forked from elastic/kibana
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
[vega] support HTML tooltips (elastic#17632)
Implement support for the richer style Vega tooltips, per elastic#17215 request. Uses [Vega tooltip plugin](https://github.com/vega/vega-tooltip) for formatting. Always enabled unless user sets `tooltips=false` flag in the `config.kibana` section of the spec. 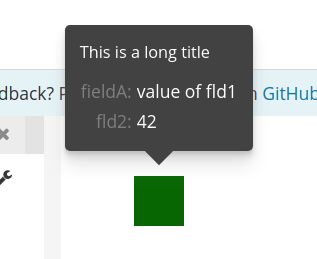 ## Test code ```js { $schema: https://vega.github.io/schema/vega/v3.json config: { kibana: { tooltips: { // always center on the mark, not mouse x,y centerOnMark: true position: top padding: 20 } } } data: [ { name: table values: [ { title: This is a long title fieldA: value of fld1 fld2: 42 } ] } ] marks: [ { from: {data: "table"} type: rect encode: { enter: { fill: {value: "elastic#60"} x: {signal: "40"} y: {signal: "40"} width: {signal: "40"} height: {signal: "40"} tooltip: {signal: "datum || null"} } } } ] } ```
- Loading branch information
Showing
11 changed files
with
298 additions
and
2 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
45 changes: 45 additions & 0 deletions
45
src/core_plugins/vega/public/__tests__/vega_tooltip_test.hjson
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,45 @@ | ||
# This graph creates a single rectangle for the whole graph, | ||
# backed by a datum with two fields - fld1 & fld2 | ||
# On mouse over, with 0 delay, it should show tooltip | ||
{ | ||
v: 1 | ||
config: { | ||
kibana: { | ||
tooltips: { | ||
// always center on the mark, not mouse x,y | ||
centerOnMark: false | ||
position: top | ||
padding: 20 | ||
} | ||
} | ||
} | ||
data: [ | ||
{ | ||
name: table | ||
values: [ | ||
{ | ||
title: This is a long title | ||
fieldA: value of fld1 | ||
fld2: 42 | ||
} | ||
] | ||
} | ||
] | ||
$schema: https://vega.github.io/schema/vega/v3.json | ||
marks: [ | ||
{ | ||
from: {data: "table"} | ||
type: rect | ||
encode: { | ||
enter: { | ||
fill: {value: "#060"} | ||
x: {signal: "0"} | ||
y: {signal: "0"} | ||
width: {signal: "width"} | ||
height: {signal: "height"} | ||
tooltip: {signal: "datum || null"} | ||
} | ||
} | ||
} | ||
] | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,79 @@ | ||
import { calculatePopoverPosition } from '@elastic/eui'; | ||
import { formatValue as createTooltipContent } from 'vega-tooltip'; | ||
import _ from 'lodash'; | ||
|
||
// Some of this code was adapted from https://github.com/vega/vega-tooltip | ||
|
||
const tooltipId = 'vega-kibana-tooltip'; | ||
|
||
/** | ||
* Simulate the result of the DOM's getBoundingClientRect() | ||
*/ | ||
function createRect(left, top, width, height) { | ||
return { | ||
left, top, width, height, | ||
x: left, y: top, right: left + width, bottom: top + height, | ||
}; | ||
} | ||
|
||
/** | ||
* The tooltip handler class. | ||
*/ | ||
export class TooltipHandler { | ||
constructor(container, view, opts) { | ||
this.container = container; | ||
this.position = opts.position; | ||
this.padding = opts.padding; | ||
this.centerOnMark = opts.centerOnMark; | ||
|
||
view.tooltip(this.handler.bind(this)); | ||
} | ||
|
||
/** | ||
* The handler function. | ||
*/ | ||
handler(view, event, item, value) { | ||
this.hideTooltip(); | ||
|
||
// hide tooltip for null, undefined, or empty string values | ||
if (value == null || value === '') { | ||
return; | ||
} | ||
|
||
const el = document.createElement('div'); | ||
el.setAttribute('id', tooltipId); | ||
el.classList.add('euiToolTipPopover', 'euiToolTip', `euiToolTip--${this.position}`); | ||
|
||
// Sanitized HTML is created by the tooltip library, | ||
// with a largue nmuber of tests, hence supressing eslint here. | ||
// eslint-disable-next-line no-unsanitized/property | ||
el.innerHTML = createTooltipContent(value, _.escape); | ||
|
||
// add to DOM to calculate tooltip size | ||
document.body.appendChild(el); | ||
|
||
// if centerOnMark numeric value is smaller than the size of the mark, use mouse [x,y] | ||
let anchorBounds; | ||
if (item.bounds.width() > this.centerOnMark || item.bounds.height() > this.centerOnMark) { | ||
// I would expect clientX/Y, but that shows incorrectly | ||
anchorBounds = createRect(event.pageX, event.pageY, 0, 0); | ||
} else { | ||
const containerBox = this.container.getBoundingClientRect(); | ||
anchorBounds = createRect( | ||
containerBox.left + view._origin[0] + item.bounds.x1, | ||
containerBox.top + view._origin[1] + item.bounds.y1, | ||
item.bounds.width(), | ||
item.bounds.height() | ||
); | ||
} | ||
|
||
const pos = calculatePopoverPosition(anchorBounds, el.getBoundingClientRect(), this.position, this.padding); | ||
|
||
el.setAttribute('style', `top: ${pos.top}px; left: ${pos.left}px`); | ||
} | ||
|
||
hideTooltip() { | ||
const el = document.getElementById(tooltipId); | ||
if (el) el.remove(); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters