-
Notifications
You must be signed in to change notification settings - Fork 8.3k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
[Actionable Observability] Integrate alert search bar on rule details…
… page (#144718) Resolves #143962 ## 📝 Summary In this PR, an alerts search bar was added to the rule details page by syncing its state to the URL. This will enable navigating to the alerts table for a specific rule with a filtered state based on active or recovered. ### Notes - Renamed alert page container to alert search bar container and used it both in alerts and rule details page (it will be responsible to sync search bar params to the URL) --> moved to a shared component - Moved AlertsStatusFilter to be a sub-component of the shared observability search bar - Allowed ObservabilityAlertSearchBar to be used both as a stand-alone component and as a wired component with syncing params to the URL (ObservabilityAlertSearchBar, ObservabilityAlertSearchbarWithUrlSync) - Set a minHeight for the Alerts and Execution tab, otherwise, the page will have extra scroll on the tab change while content is loading (very annoying!) ## 🎨 Preview 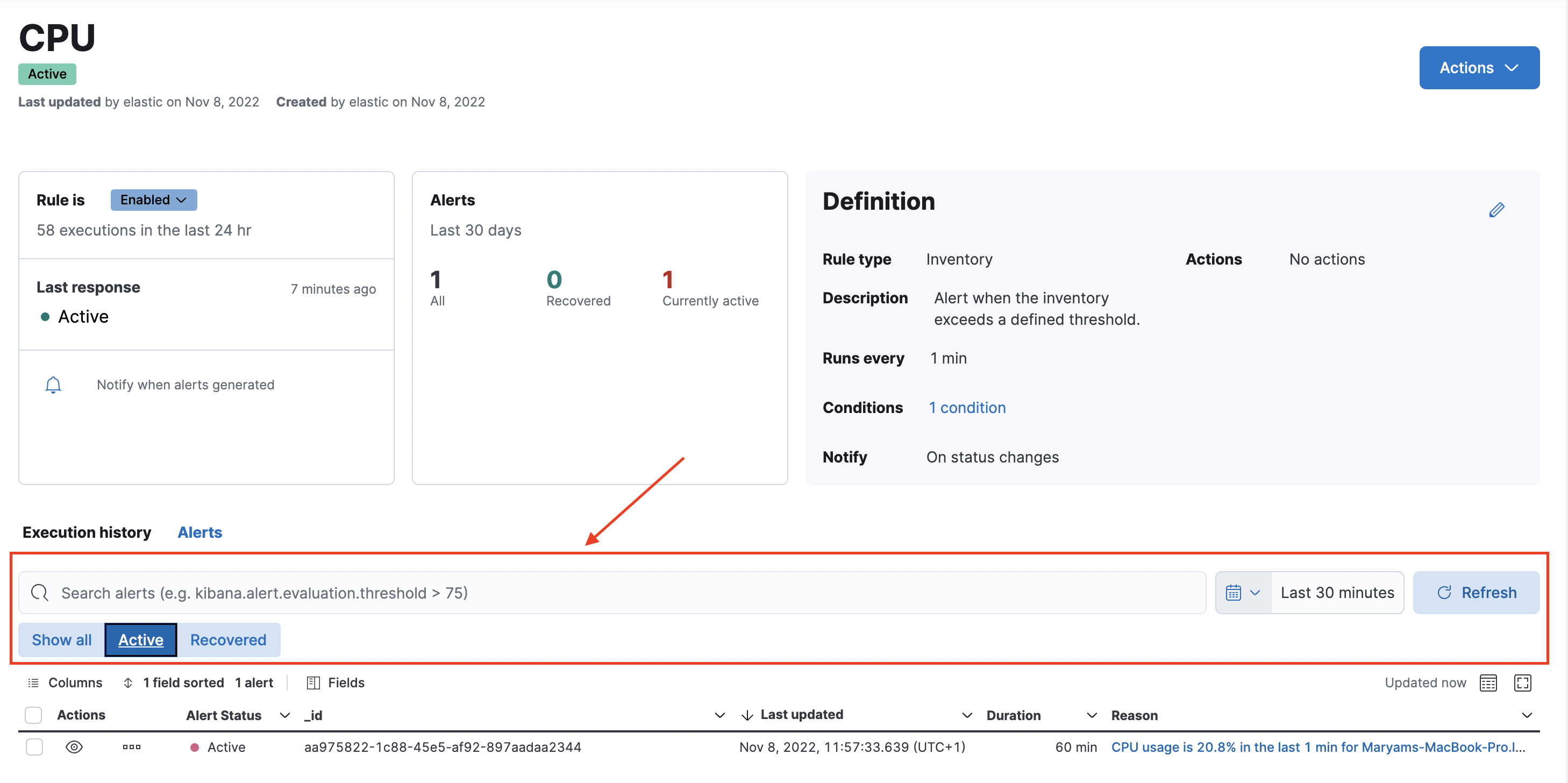 ## 🧪 How to test - Create a rule and go to the rule details page - Click on the alerts tab and change the search criteria, you should be able to see the criteria in the query parameter - Refresh the page, alerts tab should be selected and you should be able to see the filters that you applied in the previous step - As a side test, check alert search bar on alerts page as well, it should work as before Co-authored-by: kibanamachine <[email protected]>
- Loading branch information
1 parent
b1179e7
commit ef7c1a6
Showing
25 changed files
with
549 additions
and
292 deletions.
There are no files selected for viewing
124 changes: 124 additions & 0 deletions
124
x-pack/plugins/observability/public/components/shared/alert_search_bar/alert_search_bar.tsx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,124 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License | ||
* 2.0; you may not use this file except in compliance with the Elastic License | ||
* 2.0. | ||
*/ | ||
|
||
import { EuiFlexGroup, EuiFlexItem } from '@elastic/eui'; | ||
|
||
import React, { useCallback, useEffect } from 'react'; | ||
import { Query } from '@kbn/es-query'; | ||
import { useKibana } from '@kbn/kibana-react-plugin/public'; | ||
import { observabilityAlertFeatureIds } from '../../../config'; | ||
import { ObservabilityAppServices } from '../../../application/types'; | ||
import { AlertsStatusFilter } from './components'; | ||
import { ALERT_STATUS_QUERY, DEFAULT_QUERIES } from './constants'; | ||
import { AlertSearchBarProps } from './types'; | ||
import { buildEsQuery } from '../../../utils/build_es_query'; | ||
import { AlertStatus } from '../../../../common/typings'; | ||
|
||
const getAlertStatusQuery = (status: string): Query[] => { | ||
return status ? [{ query: ALERT_STATUS_QUERY[status], language: 'kuery' }] : []; | ||
}; | ||
|
||
export function AlertSearchBar({ | ||
appName, | ||
rangeFrom, | ||
setRangeFrom, | ||
rangeTo, | ||
setRangeTo, | ||
kuery, | ||
setKuery, | ||
status, | ||
setStatus, | ||
setEsQuery, | ||
queries = DEFAULT_QUERIES, | ||
}: AlertSearchBarProps) { | ||
const { | ||
data: { | ||
query: { | ||
timefilter: { timefilter: timeFilterService }, | ||
}, | ||
}, | ||
triggersActionsUi: { getAlertsSearchBar: AlertsSearchBar }, | ||
} = useKibana<ObservabilityAppServices>().services; | ||
|
||
const onStatusChange = useCallback( | ||
(alertStatus: AlertStatus) => { | ||
setEsQuery( | ||
buildEsQuery( | ||
{ | ||
to: rangeTo, | ||
from: rangeFrom, | ||
}, | ||
kuery, | ||
[...getAlertStatusQuery(alertStatus), ...queries] | ||
) | ||
); | ||
}, | ||
[kuery, queries, rangeFrom, rangeTo, setEsQuery] | ||
); | ||
|
||
useEffect(() => { | ||
onStatusChange(status); | ||
}, [onStatusChange, status]); | ||
|
||
const onSearchBarParamsChange = useCallback( | ||
({ dateRange, query }) => { | ||
timeFilterService.setTime(dateRange); | ||
setRangeFrom(dateRange.from); | ||
setRangeTo(dateRange.to); | ||
setKuery(query); | ||
setEsQuery( | ||
buildEsQuery( | ||
{ | ||
to: rangeTo, | ||
from: rangeFrom, | ||
}, | ||
query, | ||
[...getAlertStatusQuery(status), ...queries] | ||
) | ||
); | ||
}, | ||
[ | ||
timeFilterService, | ||
setRangeFrom, | ||
setRangeTo, | ||
setKuery, | ||
setEsQuery, | ||
rangeTo, | ||
rangeFrom, | ||
status, | ||
queries, | ||
] | ||
); | ||
|
||
return ( | ||
<EuiFlexGroup direction="column" gutterSize="s"> | ||
<EuiFlexItem> | ||
<AlertsSearchBar | ||
appName={appName} | ||
featureIds={observabilityAlertFeatureIds} | ||
rangeFrom={rangeFrom} | ||
rangeTo={rangeTo} | ||
query={kuery} | ||
onQueryChange={onSearchBarParamsChange} | ||
/> | ||
</EuiFlexItem> | ||
|
||
<EuiFlexItem> | ||
<EuiFlexGroup justifyContent="spaceBetween" alignItems="center"> | ||
<EuiFlexItem grow={false}> | ||
<AlertsStatusFilter | ||
status={status} | ||
onChange={(id) => { | ||
setStatus(id as AlertStatus); | ||
}} | ||
/> | ||
</EuiFlexItem> | ||
</EuiFlexGroup> | ||
</EuiFlexItem> | ||
</EuiFlexGroup> | ||
); | ||
} |
29 changes: 29 additions & 0 deletions
29
...bservability/public/components/shared/alert_search_bar/alert_search_bar_with_url_sync.tsx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License | ||
* 2.0; you may not use this file except in compliance with the Elastic License | ||
* 2.0. | ||
*/ | ||
|
||
import React from 'react'; | ||
import { | ||
alertSearchBarStateContainer, | ||
Provider, | ||
useAlertSearchBarStateContainer, | ||
} from './containers'; | ||
import { AlertSearchBar } from './alert_search_bar'; | ||
import { AlertSearchBarWithUrlSyncProps } from './types'; | ||
|
||
function InternalAlertSearchbarWithUrlSync(props: AlertSearchBarWithUrlSyncProps) { | ||
const stateProps = useAlertSearchBarStateContainer(); | ||
|
||
return <AlertSearchBar {...props} {...stateProps} />; | ||
} | ||
|
||
export function AlertSearchbarWithUrlSync(props: AlertSearchBarWithUrlSyncProps) { | ||
return ( | ||
<Provider value={alertSearchBarStateContainer}> | ||
<InternalAlertSearchbarWithUrlSync {...props} /> | ||
</Provider> | ||
); | ||
} |
44 changes: 44 additions & 0 deletions
44
...servability/public/components/shared/alert_search_bar/components/alerts_status_filter.tsx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,44 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License | ||
* 2.0; you may not use this file except in compliance with the Elastic License | ||
* 2.0. | ||
*/ | ||
|
||
import { EuiButtonGroup, EuiButtonGroupOptionProps } from '@elastic/eui'; | ||
import React from 'react'; | ||
import { ALL_ALERTS, ACTIVE_ALERTS, RECOVERED_ALERTS } from '../constants'; | ||
import { AlertStatusFilterProps } from '../types'; | ||
|
||
const options: EuiButtonGroupOptionProps[] = [ | ||
{ | ||
id: ALL_ALERTS.status, | ||
label: ALL_ALERTS.label, | ||
value: ALL_ALERTS.query, | ||
'data-test-subj': 'alert-status-filter-show-all-button', | ||
}, | ||
{ | ||
id: ACTIVE_ALERTS.status, | ||
label: ACTIVE_ALERTS.label, | ||
value: ACTIVE_ALERTS.query, | ||
'data-test-subj': 'alert-status-filter-active-button', | ||
}, | ||
{ | ||
id: RECOVERED_ALERTS.status, | ||
label: RECOVERED_ALERTS.label, | ||
value: RECOVERED_ALERTS.query, | ||
'data-test-subj': 'alert-status-filter-recovered-button', | ||
}, | ||
]; | ||
|
||
export function AlertsStatusFilter({ status, onChange }: AlertStatusFilterProps) { | ||
return ( | ||
<EuiButtonGroup | ||
legend="Filter by" | ||
color="primary" | ||
options={options} | ||
idSelected={status} | ||
onChange={onChange} | ||
/> | ||
); | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
9 changes: 9 additions & 0 deletions
9
x-pack/plugins/observability/public/components/shared/alert_search_bar/containers/index.tsx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,9 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License | ||
* 2.0; you may not use this file except in compliance with the Elastic License | ||
* 2.0. | ||
*/ | ||
|
||
export { Provider, alertSearchBarStateContainer } from './state_container'; | ||
export { useAlertSearchBarStateContainer } from './use_alert_search_bar_state_container'; |
62 changes: 62 additions & 0 deletions
62
...ns/observability/public/components/shared/alert_search_bar/containers/state_container.tsx
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,62 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License | ||
* 2.0; you may not use this file except in compliance with the Elastic License | ||
* 2.0. | ||
*/ | ||
|
||
import { | ||
createStateContainer, | ||
createStateContainerReactHelpers, | ||
} from '@kbn/kibana-utils-plugin/public'; | ||
import { AlertStatus } from '../../../../../common/typings'; | ||
import { ALL_ALERTS } from '../constants'; | ||
|
||
interface AlertSearchBarContainerState { | ||
rangeFrom: string; | ||
rangeTo: string; | ||
kuery: string; | ||
status: AlertStatus; | ||
} | ||
|
||
interface AlertSearchBarStateTransitions { | ||
setRangeFrom: ( | ||
state: AlertSearchBarContainerState | ||
) => (rangeFrom: string) => AlertSearchBarContainerState; | ||
setRangeTo: ( | ||
state: AlertSearchBarContainerState | ||
) => (rangeTo: string) => AlertSearchBarContainerState; | ||
setKuery: ( | ||
state: AlertSearchBarContainerState | ||
) => (kuery: string) => AlertSearchBarContainerState; | ||
setStatus: ( | ||
state: AlertSearchBarContainerState | ||
) => (status: AlertStatus) => AlertSearchBarContainerState; | ||
} | ||
|
||
const defaultState: AlertSearchBarContainerState = { | ||
rangeFrom: 'now-15m', | ||
rangeTo: 'now', | ||
kuery: '', | ||
status: ALL_ALERTS.status as AlertStatus, | ||
}; | ||
|
||
const transitions: AlertSearchBarStateTransitions = { | ||
setRangeFrom: (state) => (rangeFrom) => ({ ...state, rangeFrom }), | ||
setRangeTo: (state) => (rangeTo) => ({ ...state, rangeTo }), | ||
setKuery: (state) => (kuery) => ({ ...state, kuery }), | ||
setStatus: (state) => (status) => ({ ...state, status }), | ||
}; | ||
|
||
const alertSearchBarStateContainer = createStateContainer(defaultState, transitions); | ||
|
||
type AlertSearchBarStateContainer = typeof alertSearchBarStateContainer; | ||
|
||
const { Provider, useContainer } = createStateContainerReactHelpers<AlertSearchBarStateContainer>(); | ||
|
||
export { Provider, alertSearchBarStateContainer, useContainer, defaultState }; | ||
export type { | ||
AlertSearchBarStateContainer, | ||
AlertSearchBarContainerState, | ||
AlertSearchBarStateTransitions, | ||
}; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
9 changes: 9 additions & 0 deletions
9
x-pack/plugins/observability/public/components/shared/alert_search_bar/index.ts
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,9 @@ | ||
/* | ||
* Copyright Elasticsearch B.V. and/or licensed to Elasticsearch B.V. under one | ||
* or more contributor license agreements. Licensed under the Elastic License | ||
* 2.0; you may not use this file except in compliance with the Elastic License | ||
* 2.0. | ||
*/ | ||
|
||
export { AlertSearchBar as ObservabilityAlertSearchBar } from './alert_search_bar'; | ||
export { AlertSearchbarWithUrlSync as ObservabilityAlertSearchbarWithUrlSync } from './alert_search_bar_with_url_sync'; |
Oops, something went wrong.