-
Notifications
You must be signed in to change notification settings - Fork 43
API: Entities, Form Rendering, Interfaces and Actions
Violet Rails includes a flexible and powerful graph-based (JSON) object modelling framework called the API. The API consists of 5 main systems: API Namespace
, API Resource
, API Action
, API Client
and External API Connection
Used for implementing flexible schemas for CRUD (create, read, update, delete) operations.
Define your form primitives in JSON with support for: file uploads, rich text, validations and recaptcha
Once you define your form (backed by a model), you will see it rendered in real time
Form rendering can be performed by copying and pasting the CMS helper function in your HTML:
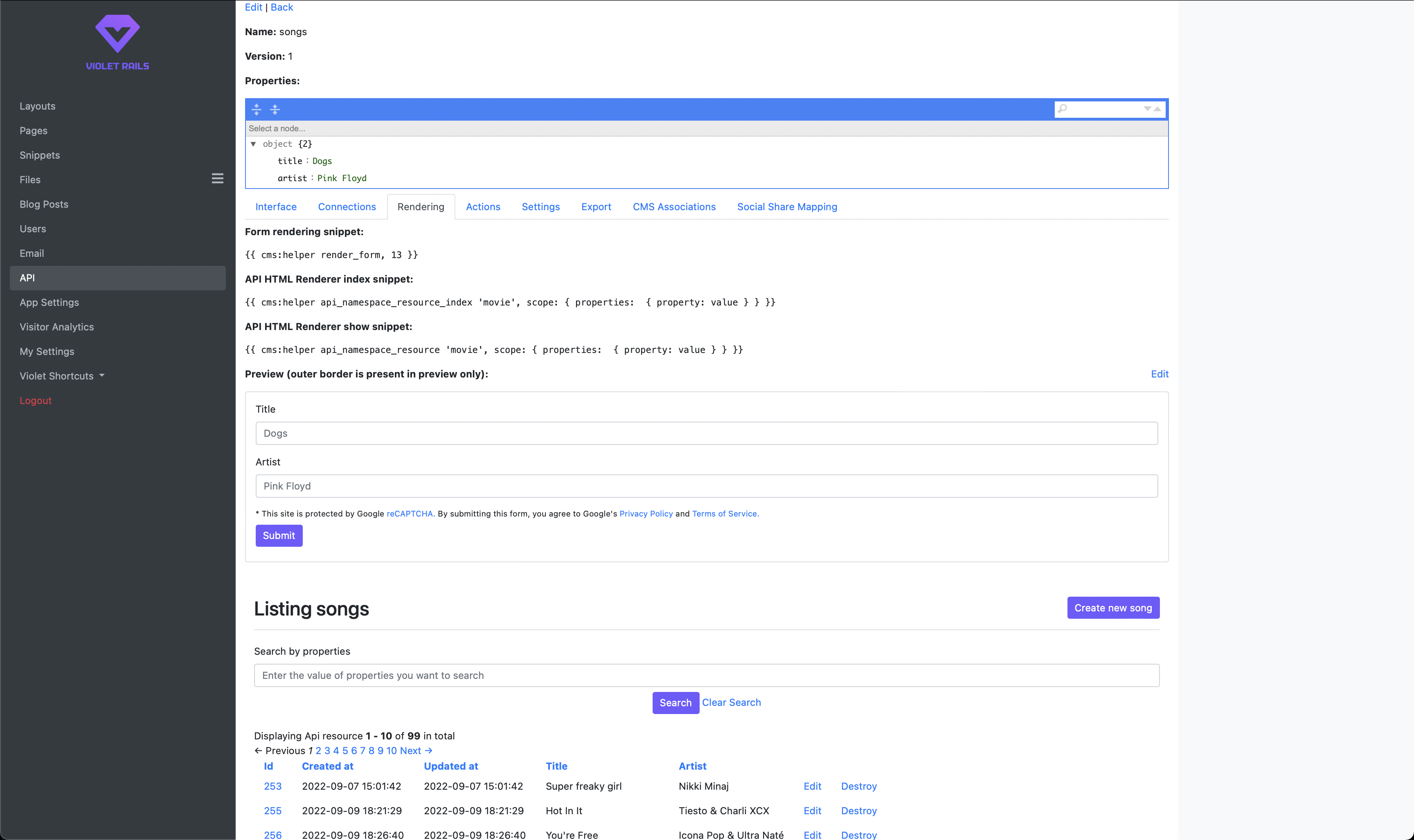
a flexible strategy for performing actions like a redirect to another page when a user submits a form, to grabbing data from an external system.
Here's a HTTP API Editor recipe for an action that sends a message to Discord when a form is submitted:
update: If you are running Violet Rails version 0.9.7+ you will need to rewrite your HTTP actions to not include the deprecated magic syntax. See more here: https://github.com/restarone/violet_rails/issues/836
To query Violet Rails from a different application, you have two options. There is a REST and GraphQL interface, both of which are available with or without authentication (if you don't use authentication you won't have write access).
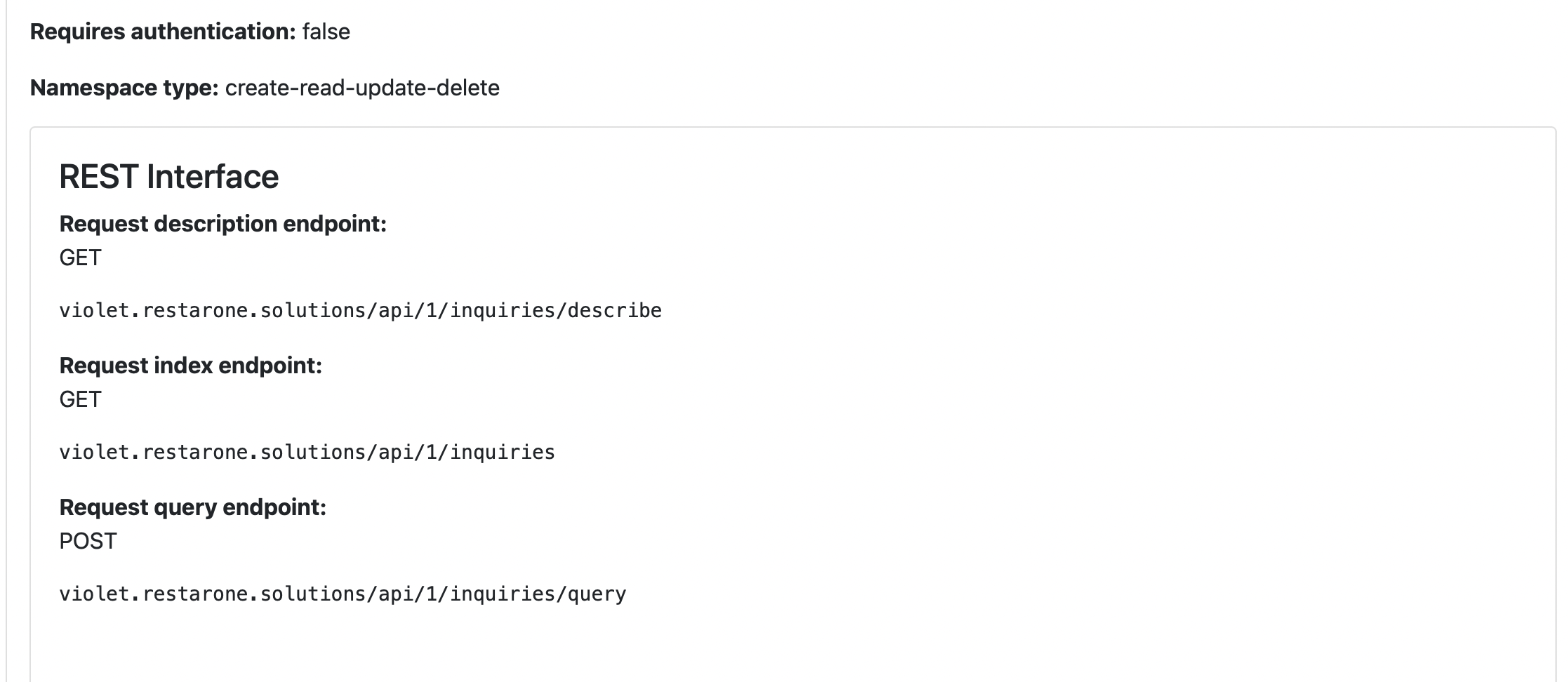
Using a combination of systems, Violet Rails is able to:
- Authenticate with any API
- Extract information from it
using a simple Ruby class defined in an External API Connection you can connect with any system using a cron or on-demand trigger. Below we have defaulted to raise error
for the model, but you can define a plain old ruby class as exemplified here
-
API Namespace
: This is the main object. Used to represent a resource, for example Users. It defines the data model along with any non-primitive attributes (file attachments, rich text etc) the model can have.
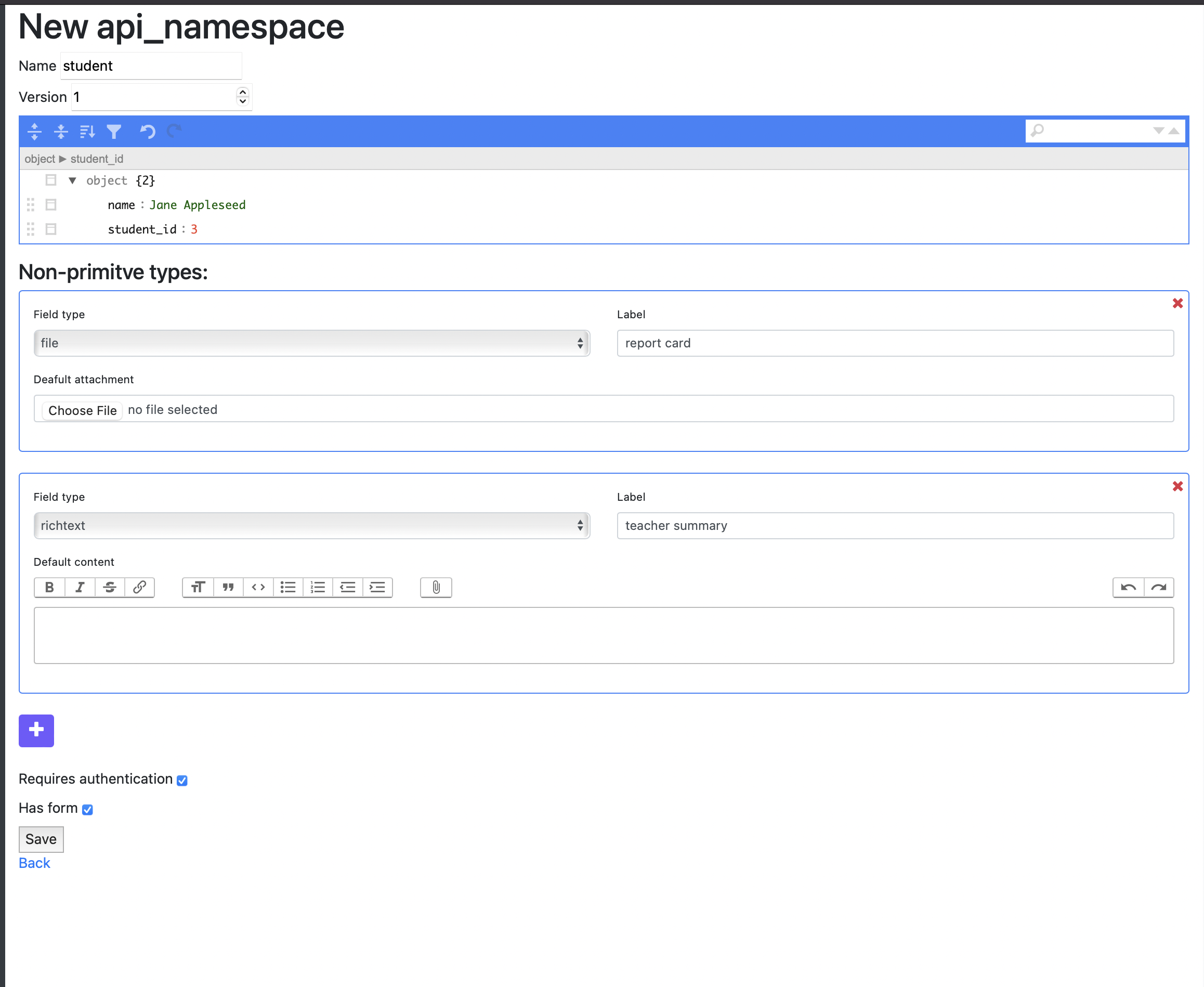
-
API Resource
: An API Namespace is the recipe for an entity. API Resource represents an instance of the entity. So a given API Namespace can have many API Resources. Eg: An API Namespace named User, will have many Users represented by a list of API Resources.
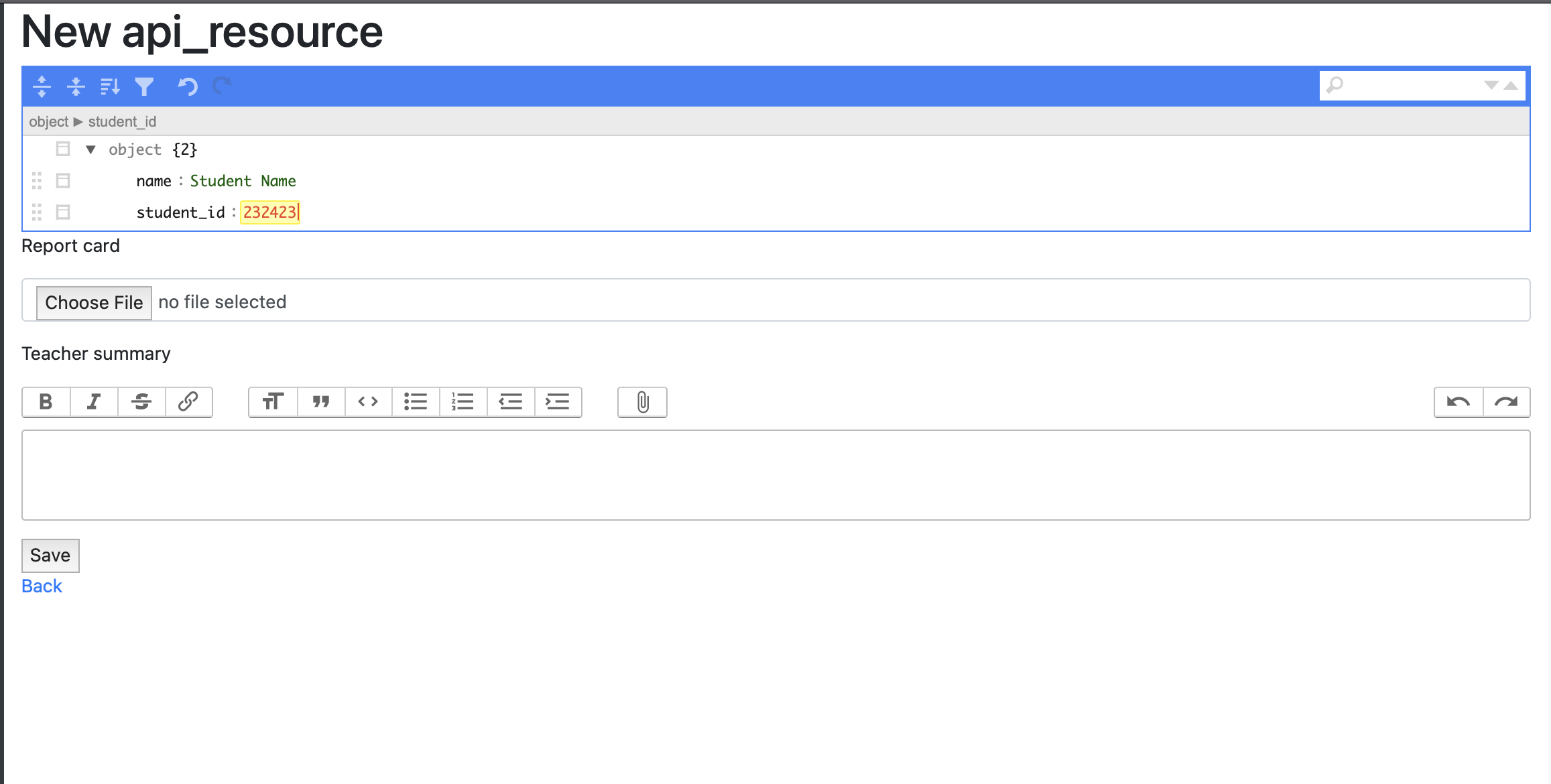
-
API Action
: is an action that that is performed when an event happens on an API Namespace or API Resource. For example, you'd want an event to be triggered when a new API Resource is created under the API Namespace User. Events can consist of: sending-- an email, a web request (to an external system), redirecting and/or serving a file (if the action is triggered in a controller).
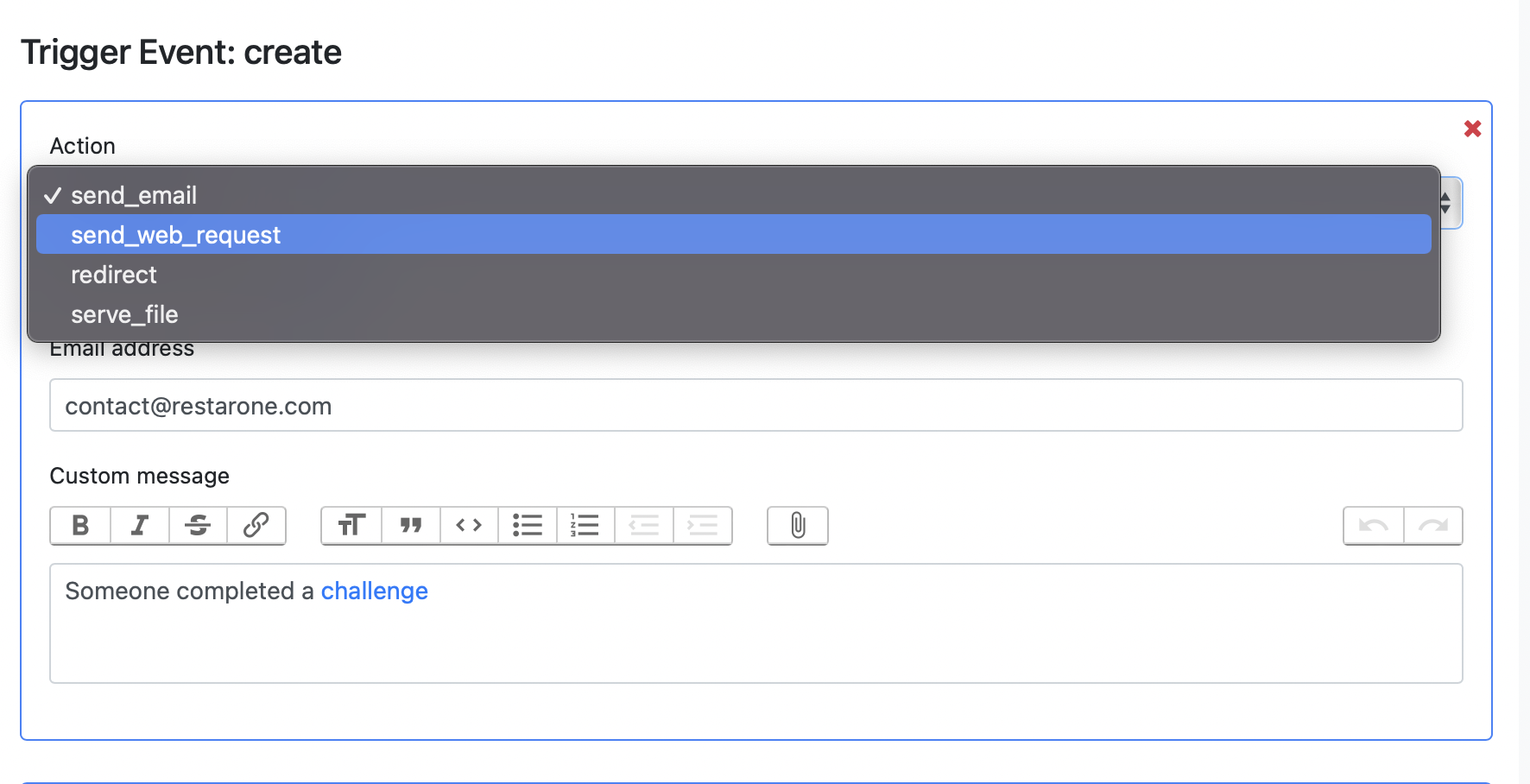
-
API Client
: is the strategy for allowing external systems to access Violet Rails API data. It involves issuing a bearer token for a given API Namespace to register a client. -
External API Connection
: a provider agnostic strategy for connecting with external systems. Includes web request retries with exponential backoff. Define your interface and connection rules in a plain Ruby class (PORO). See example in test for the requirements for a validExternal API Connection Model definition
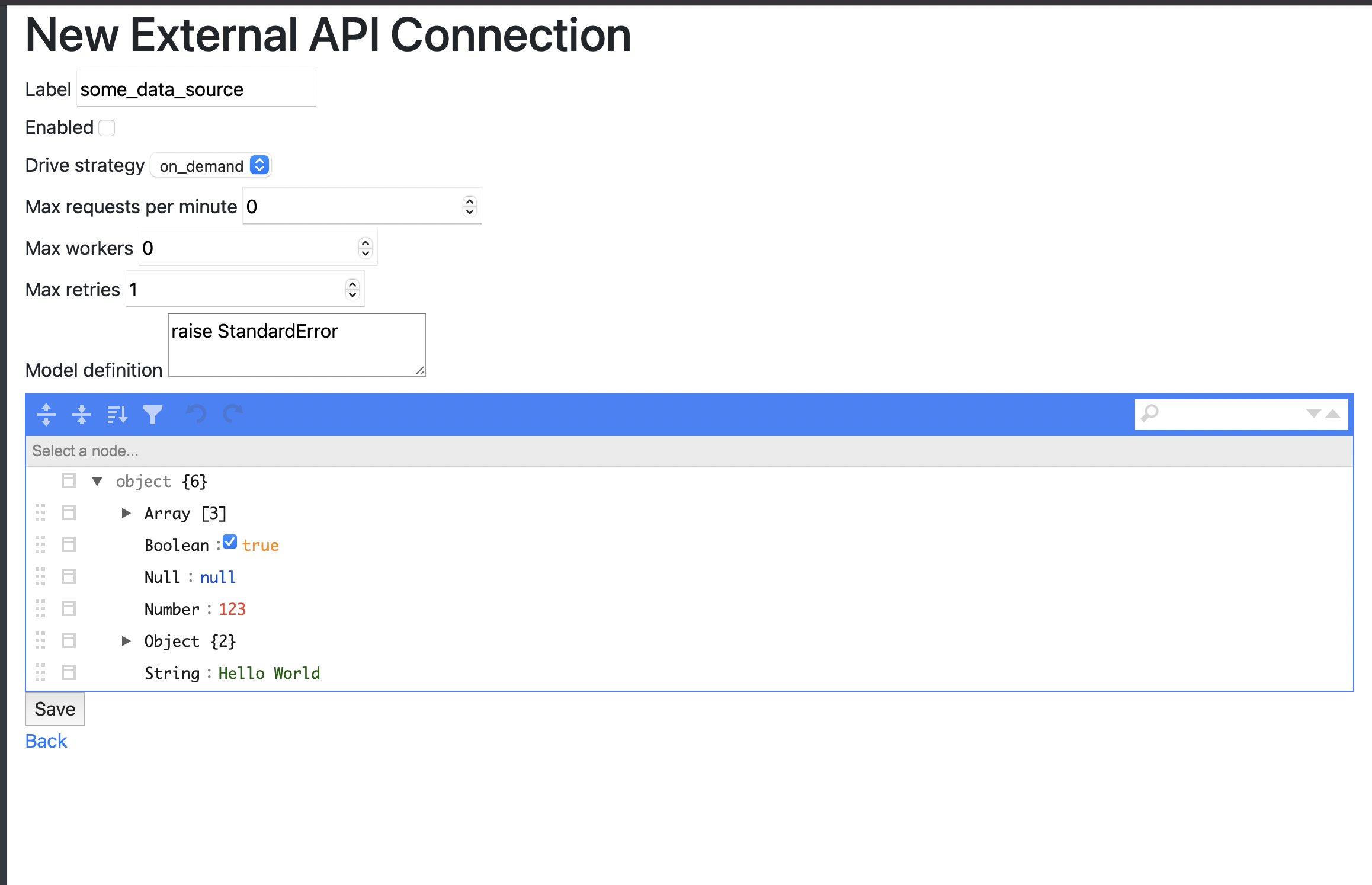
API resources of an API namespace are presented in a tabular format. Some columns are dynamically generated based on the properties of the API namespace.
For movies
API namespace below, Tags
and Title
are dynamically-generated columns:
There API system features a powerful renderer that debuted with the launch of Nikean's Psychedelic Storytelling platform: https://nikean.org
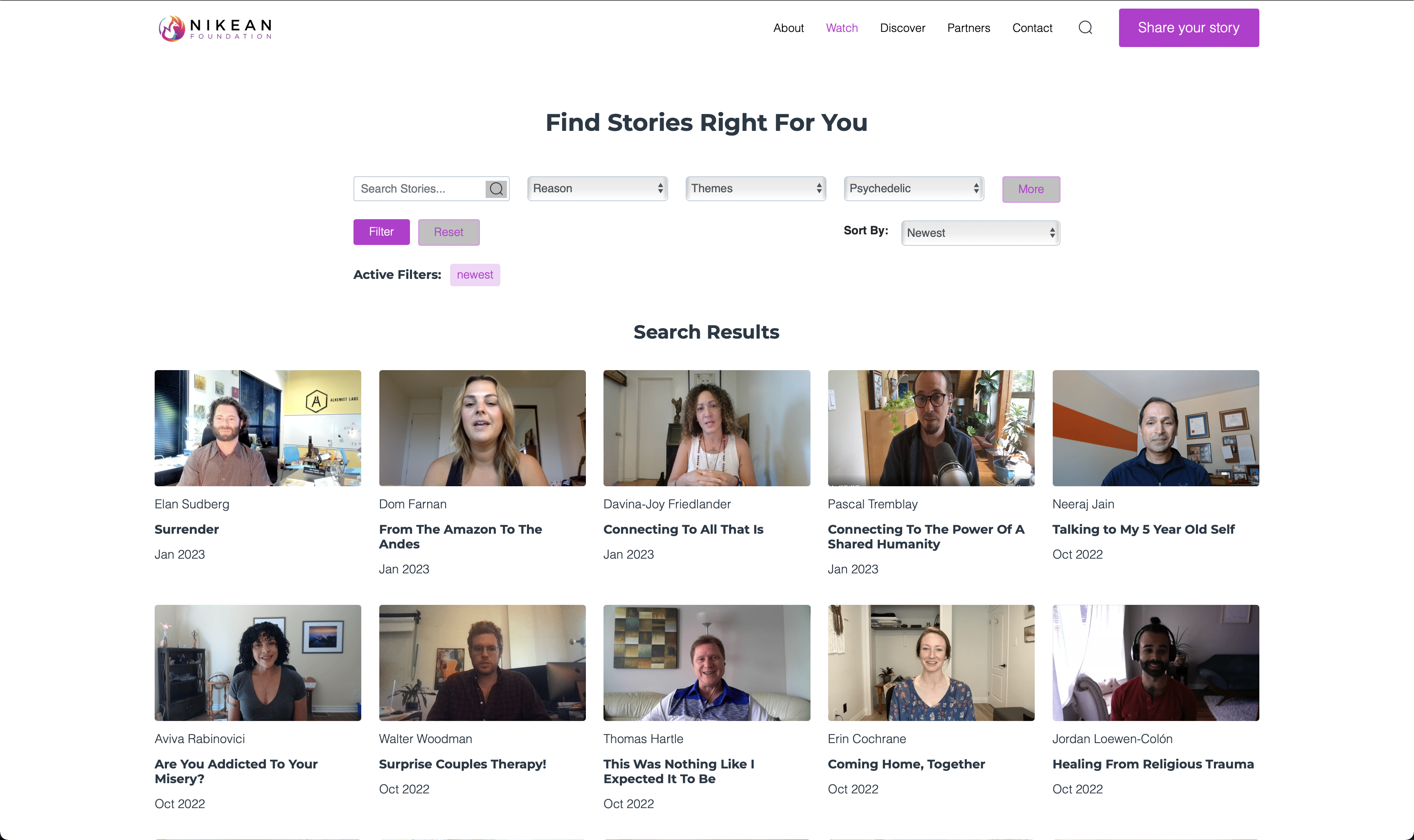
- Initial Release: https://github.com/restarone/violet_rails/releases/tag/0.9.43
- Filtering and limit added: https://github.com/restarone/violet_rails/releases/tag/0.9.44
- Associate multiple snippets: https://github.com/restarone/violet_rails/releases/tag/0.9.65
- Serve long lived file links: https://github.com/restarone/violet_rails/releases/tag/0.9.73
The HTML Renderer is connected with the API Namespace and lets you render API Resources
Create an API Namespace, or view an existing one and click on Rendering:
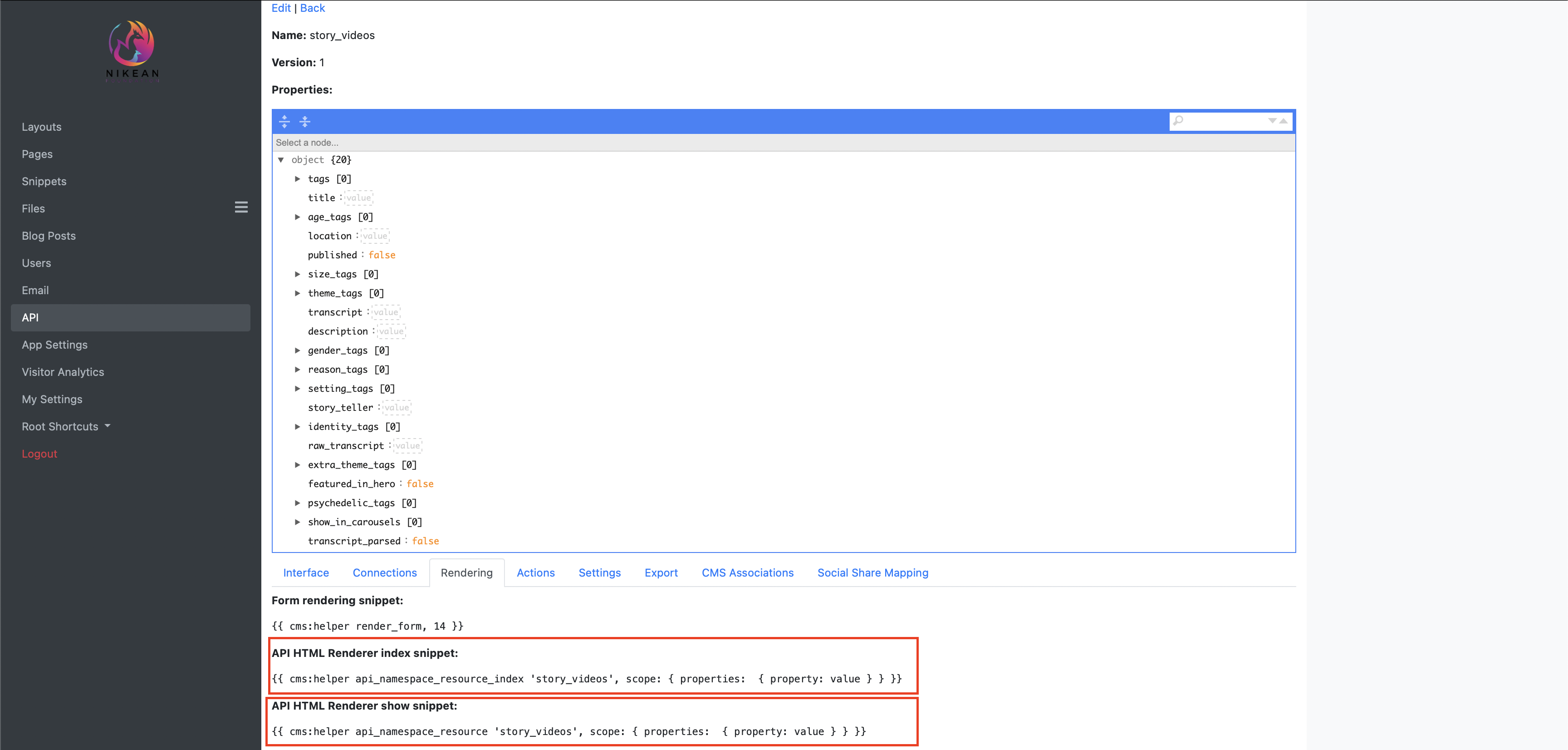
Lets dissect the snippets above:
-
{{ cms:helper api_namespace_resource_index 'story_videos', scope: { properties: { property: value } } }}
:api_namespace_resource_index
indicates that you will be rendering a list of API Resources.story_videos
refers to the slug of the API Namespace you'd like to render. Thescope
object defines the scope for the API Resources that will be rendered (eg: only showpublished: true
API Resources) -
{{ cms:helper api_namespace_resource 'story_videos', scope: { properties: { property: value } } }}
:api_namespace_resource
indicates that you will be rendering a single API Resource (typically a #show page). Thescope
object defines the scope for the API Resources that will be rendered (eg: only showpublished: true
API Resources)
Given an API Namespace named story_videos
:
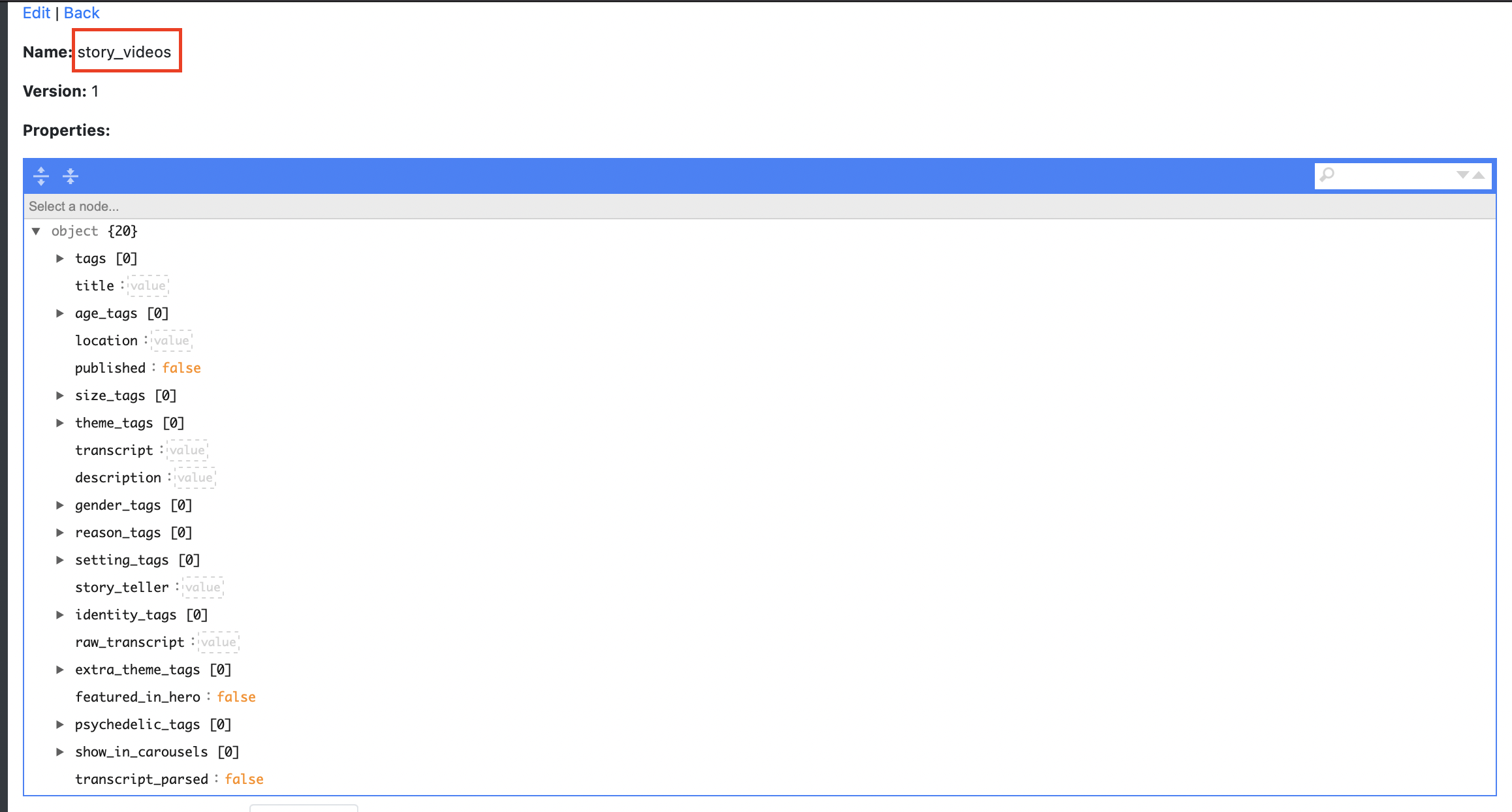
we expect the system to automatically map the Snippets used for the #index and #show views to story_videos
and story_videos-show
respectively:

The #show snippet (story_videos-show) exposes @api_resource
object so you could write ERB markup to render it however you see fit:
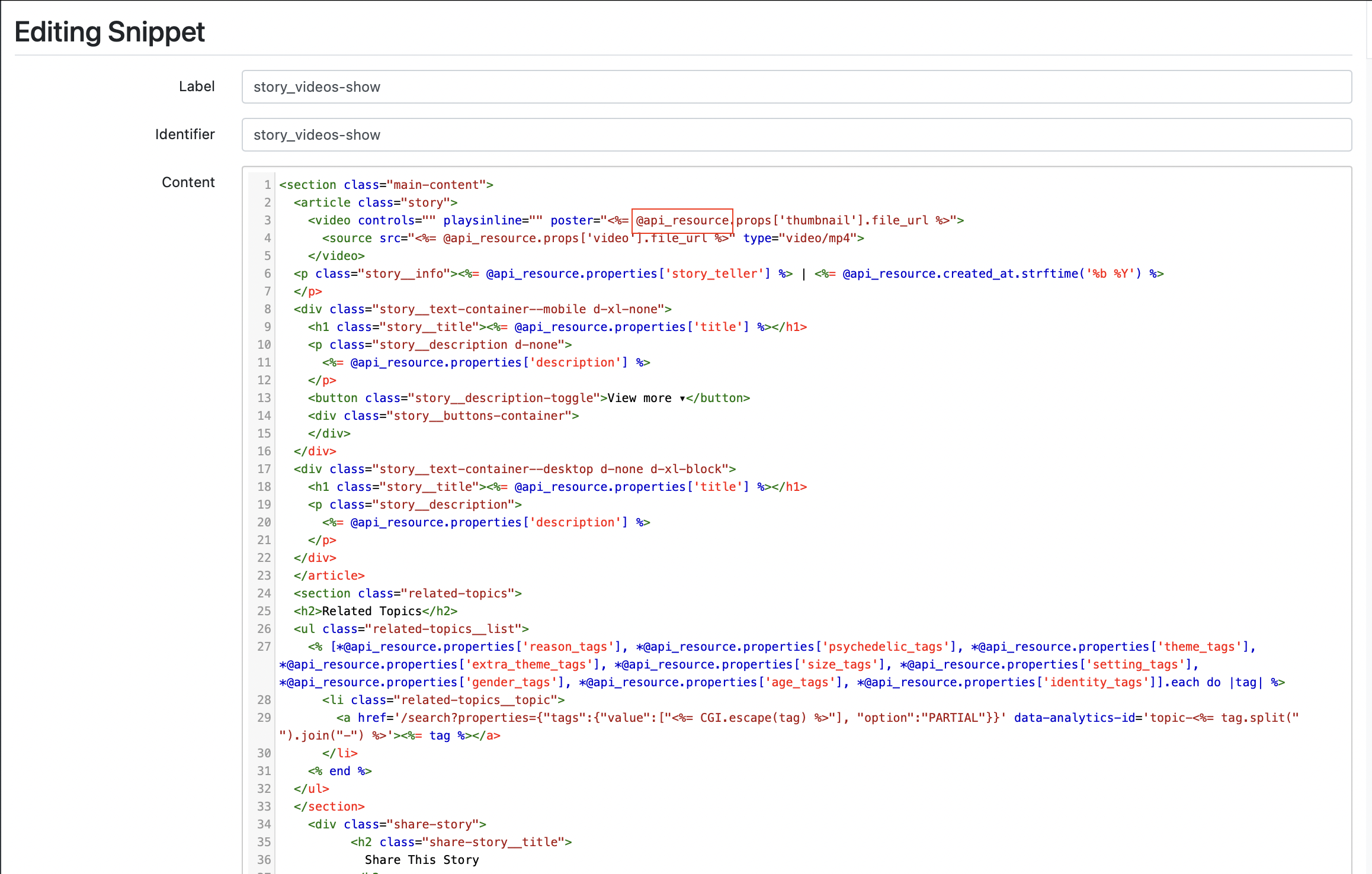
The #index snippet (story_videos) exposes @api_resources
array so you could write ERB markup to render a list of API Resources however you see fit:
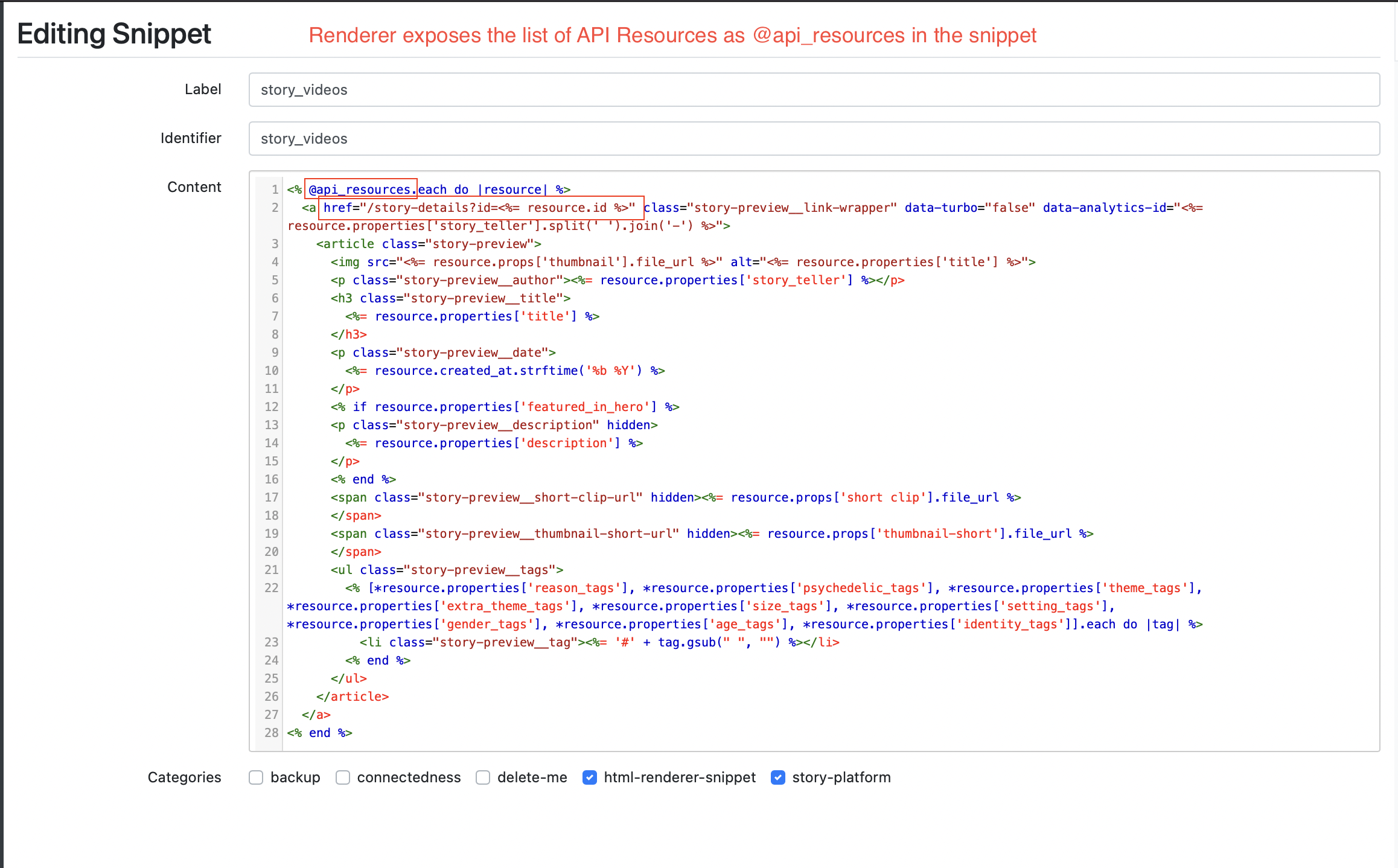

{{ cms:helper render_api_namespace_resource_index 'story_videos', scope: { properties: { published: 'true', show_in_carousels: { value: ['shorts'], option: 'PARTIAL' } } }, order: { created_at: 'DESC' }, limit: 10 }}
lets break down the above render function:
-
scope: { properties: { published: 'true', show_in_carousels: { value: ['shorts'], option: 'PARTIAL' } } }
: show published API Resources only, partial match onshorts
on the attributeshow_in_carousels
-
order: { created_at: 'DESC'
: order by created at descending -
limit: 10
: only show 10