-
Notifications
You must be signed in to change notification settings - Fork 790
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
* add Jaeger tutorial * http instr
- Loading branch information
Showing
5 changed files
with
207 additions
and
4 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,59 @@ | ||
// <copyright file="Program.cs" company="OpenTelemetry Authors"> | ||
// Copyright The OpenTelemetry Authors | ||
// | ||
// Licensed under the Apache License, Version 2.0 (the "License"); | ||
// you may not use this file except in compliance with the License. | ||
// You may obtain a copy of the License at | ||
// | ||
// http://www.apache.org/licenses/LICENSE-2.0 | ||
// | ||
// Unless required by applicable law or agreed to in writing, software | ||
// distributed under the License is distributed on an "AS IS" BASIS, | ||
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. | ||
// See the License for the specific language governing permissions and | ||
// limitations under the License. | ||
// </copyright> | ||
|
||
using System; | ||
using System.Diagnostics; | ||
using System.Net.Http; | ||
using System.Threading.Tasks; | ||
using OpenTelemetry; | ||
using OpenTelemetry.Resources; | ||
using OpenTelemetry.Trace; | ||
|
||
namespace GettingStartedJaeger; | ||
|
||
public class Program | ||
{ | ||
private static readonly ActivitySource MyActivitySource = new("OpenTelemetry.Demo.Jaeger"); | ||
|
||
public static async Task Main() | ||
{ | ||
using var tracerProvider = Sdk.CreateTracerProviderBuilder() | ||
.SetResourceBuilder(ResourceBuilder.CreateDefault().AddService( | ||
serviceName: "DemoApp", | ||
serviceVersion: "1.0.0")) | ||
.AddSource("OpenTelemetry.Demo.Jaeger") | ||
.AddHttpClientInstrumentation() | ||
.AddConsoleExporter() | ||
.AddJaegerExporter() | ||
.Build(); | ||
|
||
using var parent = MyActivitySource.StartActivity("JaegerDemo"); | ||
|
||
using (var client = new HttpClient()) | ||
{ | ||
using (var slow = MyActivitySource.StartActivity("SomethingSlow")) | ||
{ | ||
await client.GetStringAsync("https://httpstat.us/200?sleep=1000"); | ||
await client.GetStringAsync("https://httpstat.us/200?sleep=1000"); | ||
} | ||
|
||
using (var fast = MyActivitySource.StartActivity("SomethingFast")) | ||
{ | ||
await client.GetStringAsync("https://httpstat.us/301"); | ||
} | ||
} | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,133 @@ | ||
# Getting Started with Jaeger | ||
|
||
- [Export traces from the application](#export-traces-from-the-application) | ||
- [Check results in the console](#check-results-in-the-console) | ||
- [Collect and visualize traces using Jaeger](#collect-and-visualize-traces-using-jaeger) | ||
- [Final cleanup](#final-cleanup) | ||
- [Learn more](#learn-more) | ||
|
||
## Export traces from the application | ||
|
||
It is highly recommended to go over the [getting-started](../getting-started/README.md) | ||
doc before following along this document. | ||
|
||
Create a new console application and run it: | ||
|
||
```sh | ||
dotnet new console --output getting-started-jaeger | ||
cd getting-started-jaeger | ||
dotnet run | ||
``` | ||
|
||
Add reference to [Console | ||
Exporter](../../../src/OpenTelemetry.Exporter.Console/README.md), [Jaeger | ||
Exporter](../../../src/OpenTelemetry.Exporter.Jaeger/README.md) and [HttpClient | ||
Instrumentation](../../../src/OpenTelemetry.Instrumentation.Http/README.md): | ||
|
||
```sh | ||
dotnet add package OpenTelemetry.Exporter.Console | ||
dotnet add package OpenTelemetry.Exporter.Jaeger | ||
dotnet add package OpenTelemetry.Instrumentation.Http | ||
``` | ||
|
||
Now copy the code from [Program.cs](./Program.cs). | ||
|
||
### Check results in the console | ||
|
||
Run the application again and we should see the trace output from the console: | ||
|
||
```text | ||
> dotnet run | ||
Activity.TraceId: a80c920e0aabb50b547e2bb7455cfd39 | ||
Activity.SpanId: 4e45a1d51744f329 | ||
Activity.TraceFlags: Recorded | ||
Activity.ParentSpanId: 4f7e9b78c55dcfad | ||
Activity.ActivitySourceName: OpenTelemetry.Instrumentation.Http | ||
Activity.DisplayName: HTTP GET | ||
Activity.Kind: Client | ||
Activity.StartTime: 2022-05-07T02:54:25.7840762Z | ||
Activity.Duration: 00:00:01.9615540 | ||
Activity.Tags: | ||
http.method: GET | ||
http.host: httpstat.us | ||
http.url: https://httpstat.us/200?sleep=1000 | ||
http.status_code: 200 | ||
Resource associated with Activity: | ||
service.name: DemoApp | ||
service.version: 1.0.0 | ||
service.instance.id: 1b3b3a6f-be43-46b0-819a-4db1200c633d | ||
... | ||
``` | ||
|
||
Note that we have configured two exporters in the code: | ||
|
||
```csharp | ||
using var tracerProvider = Sdk.CreateTracerProviderBuilder() | ||
... | ||
.AddConsoleExporter() | ||
.AddJaegerExporter() | ||
.Build(); | ||
``` | ||
|
||
When we run the application, the `ConsoleExporter` was printing the traces on | ||
console, and the `JaegerExporter` was attempting to send the traces to Jaeger | ||
Agent via the default endpoint `udp://localhost:6831`. | ||
|
||
Since we didn't have Jaeger running, the traces received by `JaegerExporter` | ||
were simply dropped on the floor. In the next step, we are going to learn about | ||
how to use Jaeger to collect and visualize the traces. | ||
|
||
## Collect and visualize traces using Jaeger | ||
|
||
### Install and run Jaeger | ||
|
||
Download the [latest binary distribution | ||
archive](https://www.jaegertracing.io/download/) of Jaeger. | ||
|
||
After finished downloading, extract it to a local location that's easy to | ||
access. Run the `jaeger-all-in-one(.exe)` executable: | ||
|
||
```sh | ||
./jaeger-all-in-one | ||
``` | ||
|
||
Now we should be able to see the Jaeger UI at | ||
[http://localhost:16686/](http://localhost:16686/) from a web browser: | ||
|
||
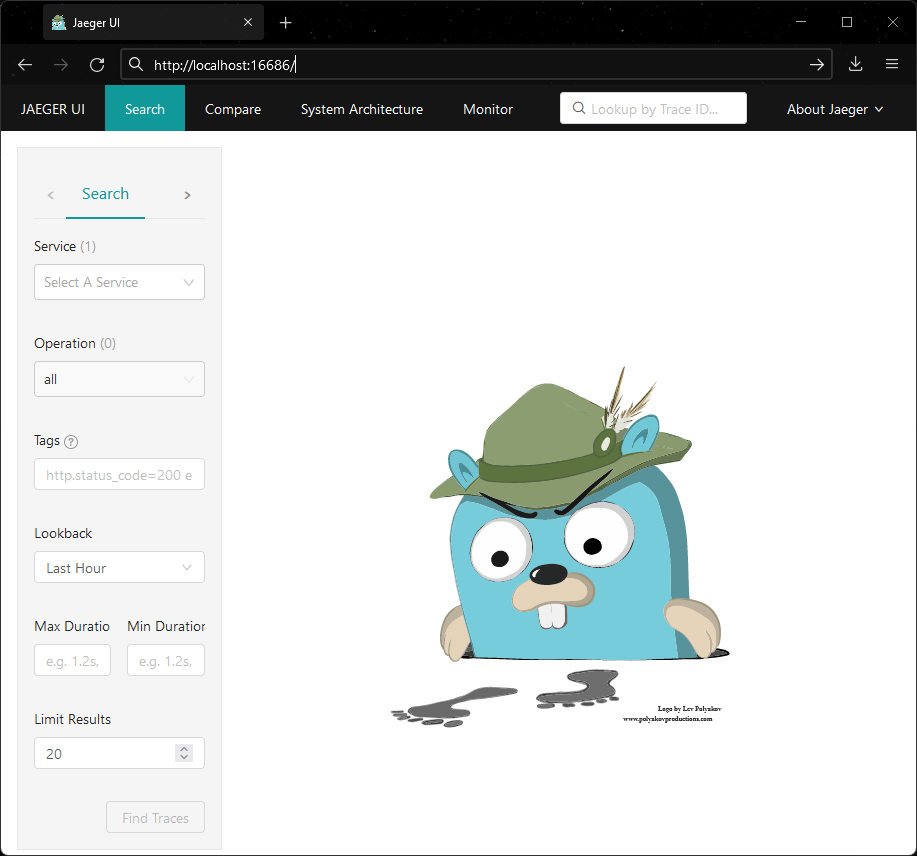 | ||
|
||
Run the application again and refresh the web page, we should be able to see the | ||
traces now: | ||
|
||
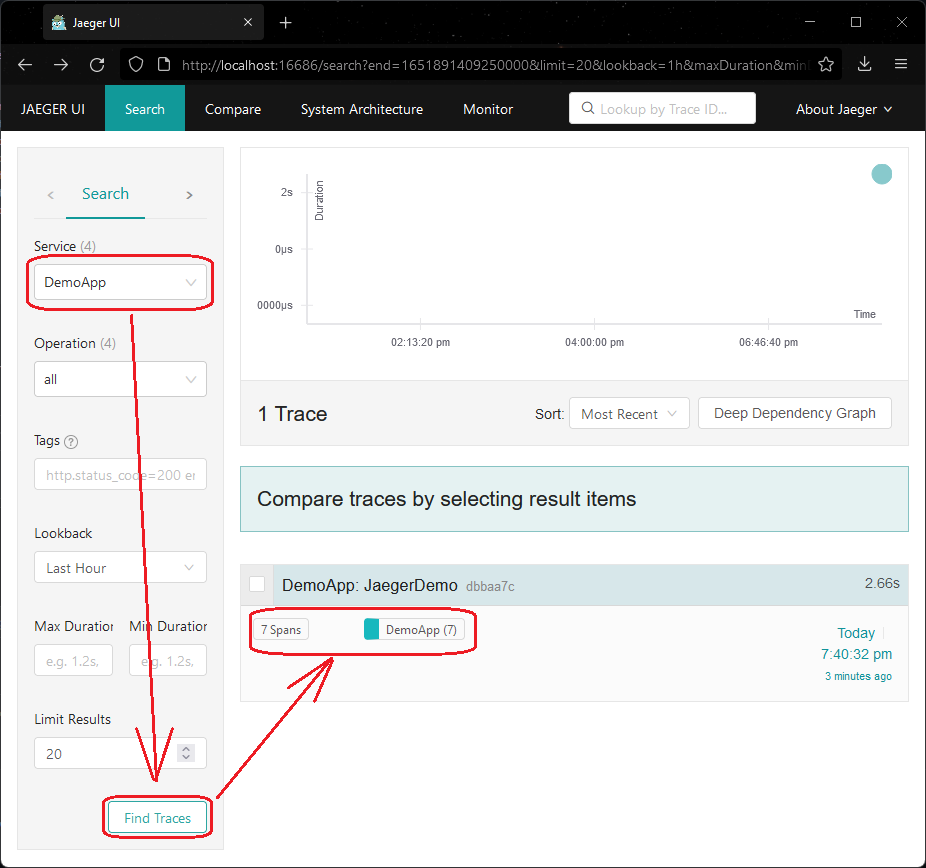 | ||
|
||
Click on the individual trace to see the [Gantt | ||
Chart](https://en.wikipedia.org/wiki/Gantt_chart): | ||
|
||
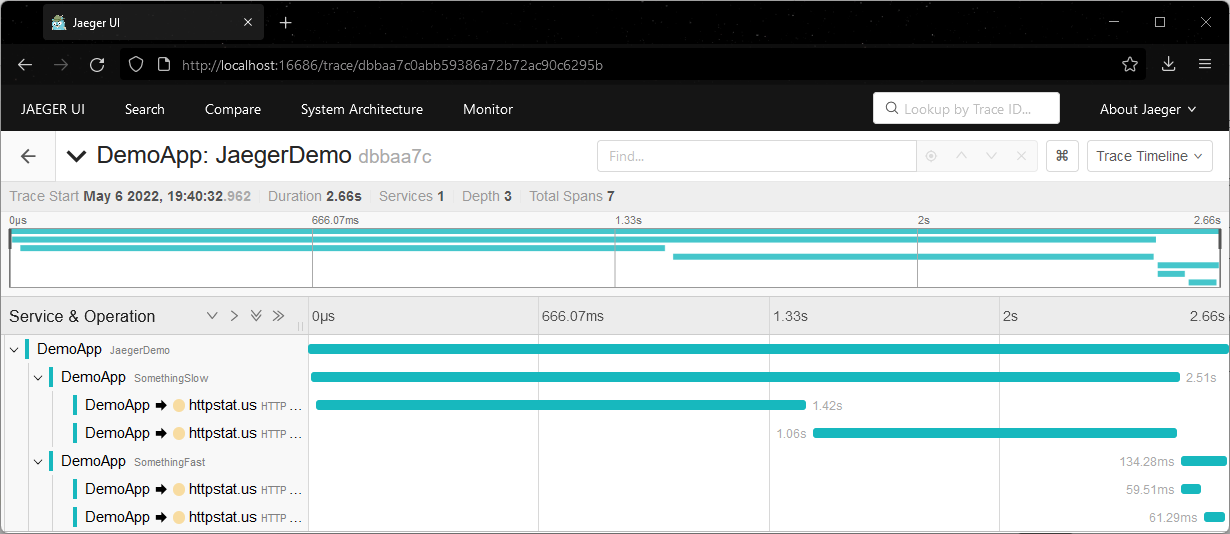 | ||
|
||
## Final cleanup | ||
|
||
In the end, remove the Console Exporter so we only have Jaeger Exporter in the | ||
final application: | ||
|
||
```csharp | ||
using var tracerProvider = Sdk.CreateTracerProviderBuilder() | ||
... | ||
// Remove Console Exporter from the final application | ||
// .AddConsoleExporter() | ||
.AddJaegerExporter() | ||
.Build(); | ||
``` | ||
|
||
```sh | ||
dotnet remove package OpenTelemetry.Exporter.Console | ||
``` | ||
|
||
## Learn more | ||
|
||
- [Jaeger Tracing](https://www.jaegertracing.io/) | ||
- [Jaeger Exporter for OpenTelemetry | ||
.NET](../../../src/OpenTelemetry.Exporter.Jaeger/README.md) |
7 changes: 7 additions & 0 deletions
7
docs/trace/getting-started-jaeger/getting-started-jaeger.csproj
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
<Project Sdk="Microsoft.NET.Sdk"> | ||
<ItemGroup> | ||
<ProjectReference Include="$(RepoRoot)\src\OpenTelemetry.Exporter.Console\OpenTelemetry.Exporter.Console.csproj" /> | ||
<ProjectReference Include="$(RepoRoot)\src\OpenTelemetry.Exporter.Jaeger\OpenTelemetry.Exporter.Jaeger.csproj" /> | ||
<ProjectReference Include="$(RepoRoot)\src\OpenTelemetry.Instrumentation.Http\OpenTelemetry.Instrumentation.Http.csproj" /> | ||
</ItemGroup> | ||
</Project> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters