-
-
Notifications
You must be signed in to change notification settings - Fork 10.6k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Show tooltip when mouse inside a cell instead of an item #6347
Comments
This seems like the same as #6250 (comment) TL;DR; There's no "one liner" way of telling is a cell is hovered in every situation because we can't always reliably get line height ahead of time. Essentially we are missing a Use |
Hi Omar, Thank you so much for the help. I'm now using constexpr ImGuiTableFlags flags = ImGuiTableFlags_Resizable | ImGuiTableFlags_Borders;
const float TEXT_BASE_HEIGHT = ImGui::GetTextLineHeightWithSpacing();
// Test tooltip.
if (ImGui::TreeNodeEx("Properties", ImGuiTreeNodeFlags_SpanFullWidth | ImGuiTreeNodeFlags_DefaultOpen))
{
if (ImGui::BeginTable("prop", 2, flags, ImVec2(0.0f, TEXT_BASE_HEIGHT * 10)))
{
ImGui::TableSetupScrollFreeze(0, 1);
ImGui::TableSetupColumn("Property");
ImGui::TableSetupColumn("Value", ImGuiTableColumnFlags_WidthStretch);
// ImGui::TableHeadersRow();
auto mouse_pos = ImGui::GetMousePos();
for (uint32_t i = 0; i < 10; ++i)
{
// Prop 1
ImGui::TableNextRow(0, TEXT_BASE_HEIGHT); // WA for knowing the height of the row.
ImGui::TableNextColumn();
ImGui::Text("Name_%u", i);
auto table = ImGui::GetCurrentTable();
assert(table);
//auto hovered_col = ImGui::TableGetHoveredColumn(); // hovered_col always equals -1
ImGuiTableColumn* column = &table->Columns[0];
// Tooltip for Prop1
if ((mouse_pos.x > column->ClipRect.Min.x)
&& (mouse_pos.x < column->ClipRect.Max.x)
&& (mouse_pos.y > table->RowPosY1)
&& (mouse_pos.y < table->RowPosY2))
{
ImGui::BeginTooltip();
ImGui::Text("Tooltip on name %u", i);
ImGui::EndTooltip();
}
// Value 1
ImGui::TableNextColumn();
ImGui::Text("Value_%u", i);
column = &table->Columns[1];
// Tooltip for Value 1
if ((mouse_pos.x > column->ClipRect.Min.x)
&& (mouse_pos.x < column->ClipRect.Max.x)
&& (mouse_pos.y > table->RowPosY1)
&& (mouse_pos.y < table->RowPosY2))
{
ImGui::BeginTooltip();
ImGui::Text("Tooltip on value %u", i);
ImGui::EndTooltip();
}
}
ImGui::EndTable();
}
ImGui::TreePop();
} |
You are right. It's must too late yesterday :) Both So here is the code: constexpr ImGuiTableFlags flags = ImGuiTableFlags_Resizable | ImGuiTableFlags_Borders;
const float TEXT_BASE_HEIGHT = ImGui::GetTextLineHeightWithSpacing();
// Test tooltip.
if (ImGui::TreeNodeEx("Properties", ImGuiTreeNodeFlags_SpanFullWidth | ImGuiTreeNodeFlags_DefaultOpen))
{
if (ImGui::BeginTable("prop", 2, flags, ImVec2(0.0f, TEXT_BASE_HEIGHT * 10)))
{
ImGui::TableSetupScrollFreeze(0, 1);
ImGui::TableSetupColumn("Property");
ImGui::TableSetupColumn("Value", ImGuiTableColumnFlags_WidthStretch);
// ImGui::TableHeadersRow();
// Function returns if current cell is hovered.
auto cell_hovered = []()
{
auto mouse_pos = ImGui::GetMousePos();
auto table = ImGui::GetCurrentTable();
return ImGui::TableGetCellBgRect(table, table->CurrentColumn).Contains(mouse_pos);
};
for (uint32_t i = 0; i < 10; ++i)
{
// Name
ImGui::TableNextRow(0, TEXT_BASE_HEIGHT); // WA for knowing the height of the row.
ImGui::TableNextColumn();
ImGui::Text("Name_%u", i);
// Tooltip for name
if (cell_hovered())
{
ImGui::BeginTooltip();
ImGui::Text("Tooltip on Name %u", i);
ImGui::EndTooltip();
}
// Value
ImGui::TableNextColumn();
ImGui::Text("Value_%u", i);
// Tooltip for value
if (cell_hovered())
{
ImGui::BeginTooltip();
ImGui::Text("Tooltip on Value %u", i);
ImGui::EndTooltip();
}
}
ImGui::EndTable();
}
ImGui::TreePop();
} |
So here comes the next question... how to handle the delay for the tooltip. |
Closing this.
Where I'll post here and #6250 if I decide to add a |
You will need to maintain the timer yourself. As per #1485 (comment) while we added delay support in |
Hi Omar,
Thanks again for the amazing UI solution!
I'm using Table APIs and trying to to show a tooltip for each of the cells, however, if using
ImGui::IsItemHovered()
as the condition, the hovering rect is the actual item rect and if the item is very small, e.g. one character, it's very hard to have the tooltip showed.Is there a way to trigger the tooltip in the range of a cell size?
Screen record
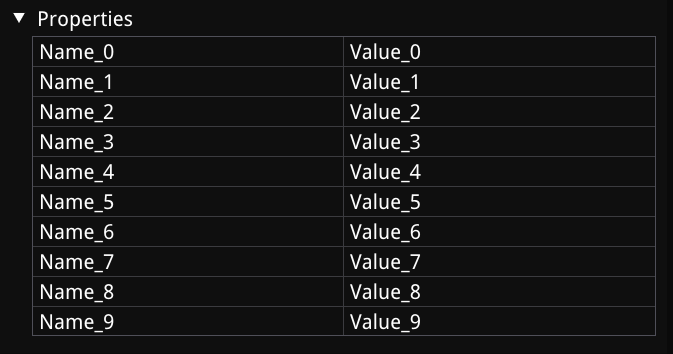
Version/Branch of Dear ImGui:
Code to reproduce the issue
Best regards,
Kai
The text was updated successfully, but these errors were encountered: