Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
[Logs UI] Refactor Time and Position log stream state (#149052)
## Summary Closes #145137 Initially this issue was just going to include moving the time context into the query state machine. However, this actually made working with the dependant log position code a lot harder. As such the log position code has also been moved to it's own state machine. ## 🕵️♀️ Reviewer hints and notable changes - There are some comments left inline (*previous logic* notes might be useful) - There is now a new machine for dealing with Log Position state (target position, latest position, visible positions). - Time based context (time range, timestamps, refresh interval) is now moved to the query machine (this will also make dealing with saved queries easier). - The page state machine is the only machine that the UI interacts with (either reading context or sending events). The page state machine co-ordinates forwarding necessary events to other internal machines. - Ensure relevant notifications reach their targets, e.g. when time is changed, positions should also update. - [There is some documentation regarding URL state and precedence](f9ca0f7). - `updateContextInUrl` now always sets the full URL-relevant context in the URL when called (since the `urlStateStorage.set()` call replaces the whole key). - Within the Log Stream Query state machine the `initialized` state node is now modelled as a parallel state node, this is so `query` and `time` can act independently (time needs to handle things like the refresh interval, for example). ## 🕹 Testing (Just some ideas) - Can the time range be changed? - Can the refresh interval be changed? - Is state synchronised to the URL and to relevant Kibana services (time filter service etc)? - When streaming is enabled, are requests dispatched correctly on an interval? - Do positions update correctly whilst interacting with the stream? (scrolling etc) - Does the backwards compatibility of initialising from the URL work? ## 🎨 State machine diagrams ### Log stream page 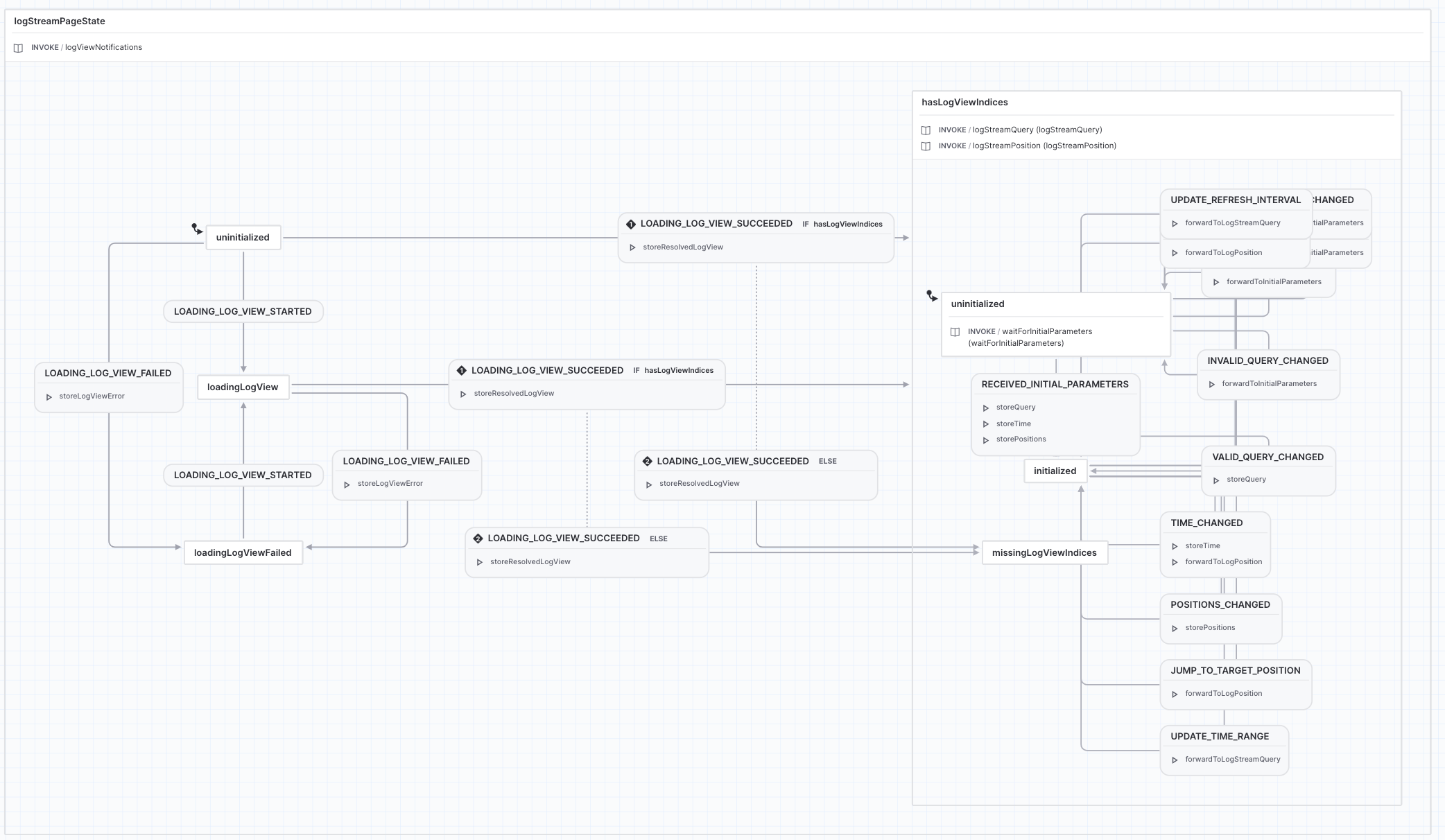 ### Log stream query 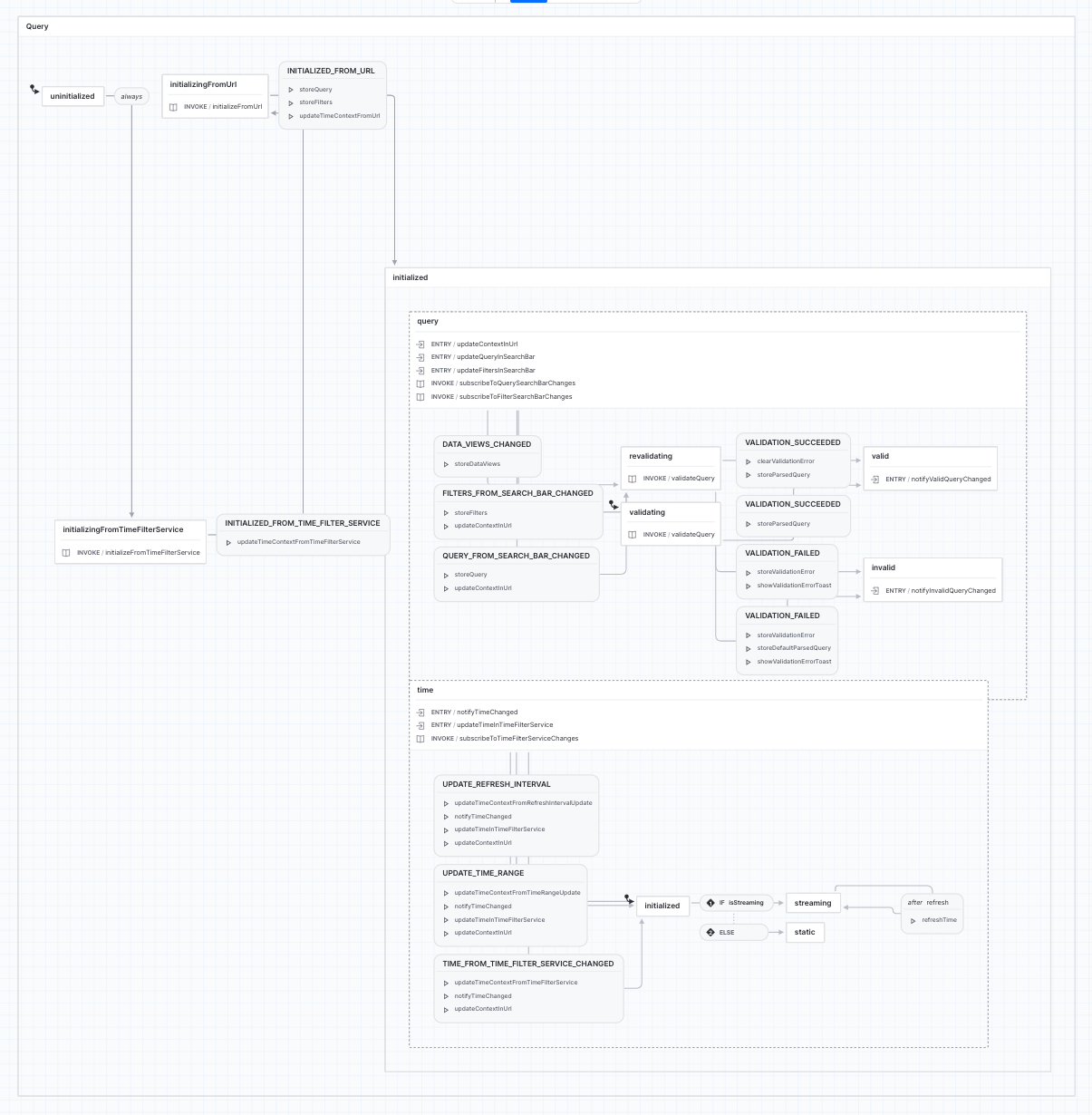 ### Log stream position 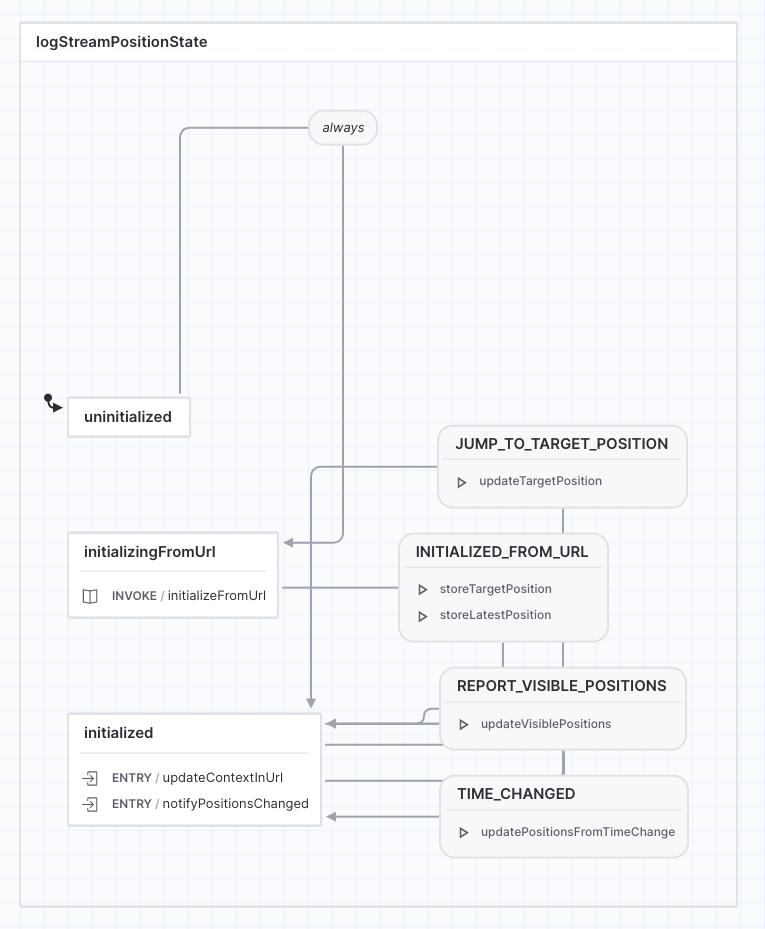 ##⚠️ Warnings - [There is a known bug with streaming](#136159 (comment)). - [There is a known issue with a console error](#149052 (comment)) --------- Co-authored-by: Felix Stürmer <[email protected]>
- Loading branch information