-
Notifications
You must be signed in to change notification settings - Fork 17
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #1 from datastructures-js/development
v1.0.0
- Loading branch information
Showing
16 changed files
with
1,135 additions
and
2 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,23 @@ | ||
{ | ||
"rules": { | ||
max-len: [ | ||
"error", | ||
{ | ||
"code": 80, | ||
"ignoreComments": true | ||
} | ||
], | ||
"comma-dangle": [ | ||
"error", | ||
{ | ||
"functions": "ignore" | ||
} | ||
], | ||
"class-methods-use-this":0 | ||
}, | ||
"env": { | ||
"mocha": true, | ||
"node": true | ||
}, | ||
"extends": ["airbnb-base"] | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,3 @@ | ||
node_modules | ||
coverage | ||
.DS_Store |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,5 @@ | ||
.git* | ||
test | ||
.travis.yml | ||
Gruntfile.js | ||
coverage/ |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,10 @@ | ||
language: node_js | ||
node_js: | ||
- "10" | ||
- "11" | ||
- "12" | ||
install: | ||
- npm install -g grunt-cli | ||
- npm install | ||
script: | ||
- grunt build |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
# Changelog | ||
All notable changes to this project will be documented in this file. | ||
|
||
The format is based on [Keep a Changelog](https://keepachangelog.com/en/1.0.0/), | ||
and this project adheres to [Semantic Versioning](https://semver.org/spec/v2.0.0.html). | ||
|
||
## [Unreleased] | ||
|
||
## [1.0.0] - 2019-12-15 | ||
### Added | ||
- initial release |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,27 @@ | ||
module.exports = (grunt) => { | ||
grunt.initConfig({ | ||
eslint: { | ||
src: ['src/*.js', 'test/*.test.js'] | ||
}, | ||
mochaTest: { | ||
files: ['test/*.test.js'] | ||
}, | ||
mocha_istanbul: { | ||
coverage: { | ||
src: 'test', | ||
options: { | ||
mask: '*.test.js' | ||
} | ||
} | ||
} | ||
}); | ||
|
||
grunt.loadNpmTasks('grunt-eslint'); | ||
grunt.loadNpmTasks('grunt-mocha-test'); | ||
grunt.loadNpmTasks('grunt-mocha-istanbul'); | ||
|
||
grunt.registerTask('lint', ['eslint']); | ||
grunt.registerTask('test', ['mochaTest']); | ||
grunt.registerTask('coverage', ['mocha_istanbul']); | ||
grunt.registerTask('build', ['lint', 'coverage']); | ||
}; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,21 @@ | ||
The MIT License (MIT) | ||
|
||
Copyright (c) 2019 Eyas Ranjous <[email protected]> | ||
|
||
Permission is hereby granted, free of charge, to any person obtaining a copy | ||
of this software and associated documentation files (the "Software"), to deal | ||
in the Software without restriction, including without limitation the rights | ||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
copies of the Software, and to permit persons to whom the Software is | ||
furnished to do so, subject to the following conditions: | ||
|
||
The above copyright notice and this permission notice shall be included in all | ||
copies or substantial portions of the Software. | ||
|
||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
SOFTWARE. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,2 +1,191 @@ | ||
# heap | ||
Heap (min & max) implementation in javascript | ||
# @datastructures-js/heap | ||
[](https://travis-ci.org/datastructures-js/heap) | ||
[](https://www.npmjs.com/package/@datastructures-js/heap) | ||
[](https://www.npmjs.com/package/@datastructures-js/heap) [](https://www.npmjs.com/package/@datastructures-js/heap) | ||
|
||
a complete javascript implementation for the Min/Max Heap data structures & Heap Sort algorithm. | ||
|
||
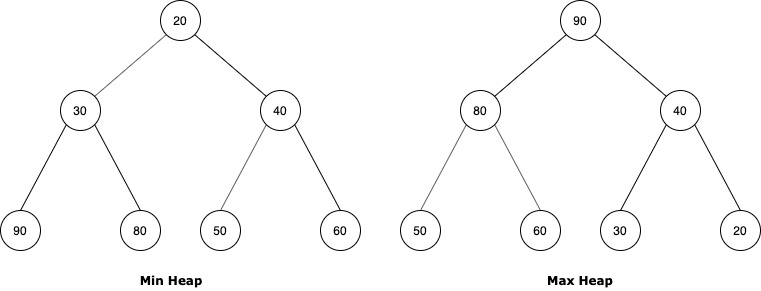 | ||
|
||
## install | ||
```sh | ||
npm install --save @datastructures-js/heap | ||
``` | ||
|
||
## API | ||
|
||
### require | ||
```js | ||
const { MinHeap, MaxHeap } = require('@datastructures-js/heap'); | ||
``` | ||
|
||
### import | ||
```js | ||
import { MinHeap, MaxHeap } from '@datastructures-js/heap'; | ||
``` | ||
|
||
### create a heap | ||
|
||
#### new | ||
creates an empty heap. | ||
|
||
```js | ||
const minHeap = new MinHeap(); | ||
|
||
const maxHeap = new MaxHeap(); | ||
``` | ||
|
||
#### .heapify(list) | ||
converts an array of objects to a heap. | ||
|
||
the function can read a list elements that are **number**, **string**, or a **serialized heap node** like `{ key: 10, value: { someProp: 'someVal' } }`. | ||
|
||
```js | ||
const list = [ | ||
50, | ||
80, | ||
{ key: 30, value: 'something' }, | ||
90, | ||
{ key: 60, value: null }, | ||
40, | ||
{ key: 20, value: { name: 'test' } } | ||
]; | ||
|
||
const minHeap = MinHeap.heapify(list); | ||
|
||
const maxHeap = MaxHeap.heapify(list); | ||
``` | ||
|
||
### .insert(key, value) | ||
insert a node into the heap. | ||
|
||
**key** can be a **number** or a **string** | ||
|
||
**value** can be any **object** type. | ||
|
||
a heap node is created as an instance of **NodeHeap**. | ||
|
||
```js | ||
const minHeap = new MinHeap(); | ||
|
||
const maxHeap = new MaxHeap(); | ||
|
||
minHeap.insert(50); | ||
minHeap.insert(80); | ||
minHeap.insert(30, 'something'); | ||
minHeap.insert(90); | ||
minHeap.insert(60, null); | ||
minHeap.insert(40); | ||
minHeap.insert(20, { name: 'test' }); | ||
|
||
maxHeap.insert(50); | ||
maxHeap.insert(80); | ||
maxHeap.insert(30, 'something'); | ||
maxHeap.insert(90); | ||
maxHeap.insert(60, null); | ||
maxHeap.insert(40); | ||
maxHeap.insert(20, { name: 'test' }); | ||
``` | ||
|
||
### HeapNode | ||
returned with .root() & .extractRoot() functions. It implements the following interface | ||
|
||
#### .getKey() | ||
returns the node's key (number or string) that is used to compare with other. | ||
|
||
#### .getValue() | ||
returns the value that is associated with the key. | ||
|
||
#### .serialize() | ||
returns an object literal of key/value of the node. | ||
|
||
### .root() | ||
returns (peeks) the root node without removing it. | ||
|
||
```js | ||
const min = minHeap.root(); | ||
console.log(min.getKey()); // 20 | ||
console.log(min.getValue()); // { name: 'test' } | ||
console.log(min.serialize()); // { key: 20, value: { name: 'test' } } | ||
|
||
const max = maxHeap.root(); | ||
console.log(max.getKey()); // 90 | ||
console.log(max.getValue()); // undefined | ||
console.log(max.serialize()); // { key: 90, value: undefined } | ||
``` | ||
|
||
### .extractRoot() | ||
returns and remove the root node in the heap. | ||
|
||
```js | ||
const min = minHeap.extractRoot(); | ||
console.log(min.getKey()); // 20 | ||
console.log(min.getValue()); // { name: 'test' } | ||
console.log(min.serialize()); // { key: 20, value: { name: 'test' } } | ||
console.log(minHeap.root().getKey()); // 30 | ||
|
||
const max = maxHeap.extractRoot(); | ||
console.log(max.getKey()); // 90 | ||
console.log(max.getValue()); // undefined | ||
console.log(max.serialize()); // { key: 20, value: undefined } | ||
console.log(maxHeap.root().getKey()); // 80 | ||
``` | ||
|
||
### .size() | ||
returns the number of nodes in the heap. | ||
|
||
```js | ||
console.log(minHeap.size()); // 6 | ||
console.log(maxHeap.size()); // 6 | ||
``` | ||
|
||
### .clone() | ||
creates a shallow copy of a heap by slicing the nodes array and passing it to a new heap instance. | ||
|
||
```js | ||
const minHeapClone = minHeap.clone(); | ||
minHeapClone.extractRoot(); | ||
|
||
console.log(minHeapClone.root().getKey()); // 40 | ||
console.log(minHeap.root().getKey()); // 30 | ||
``` | ||
|
||
### .sort() | ||
implements Heap Sort and sorts a **Max Heap in ascneding order** or a **Min Heap in descending order**. | ||
|
||
calling .sort() directly on a heap will mutate its nodes location. To avoid that, you can sort a shallow copy of the heap. | ||
|
||
```js | ||
const sortedAsc = maxHeap.clone().sort(); // does not mutate the heap structure | ||
const sortedDesc = minHeap.clone().sort(); // does not mutate the heap structure | ||
``` | ||
|
||
If you are using this npm for the purpose of sorting a list of elements using Heap Sort, you can do it like this | ||
|
||
```js | ||
const sortedAsc = MaxHeap.heapify(unsortedList).sort(); | ||
const sortedDesc = MinHeap.heapify(unsortedList).sort(); | ||
``` | ||
|
||
### .clear() | ||
clears the nodes in the heap | ||
|
||
```js | ||
minHeap.clear(); | ||
maxHeap.clear(); | ||
|
||
console.log(minHeap.size()); // 0 | ||
console.log(minHeap.root()); // null | ||
|
||
console.log(maxHeap.size()); // 0 | ||
console.log(maxHeap.root()); // null | ||
``` | ||
|
||
## Build | ||
lint + tests | ||
``` | ||
grunt build | ||
``` | ||
|
||
## License | ||
The MIT License. Full License is [here](https://github.com/datastructures-js/heap/blob/master/LICENSE) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,7 @@ | ||
const MinHeap = require('./src/minHeap'); | ||
const MaxHeap = require('./src/maxHeap'); | ||
|
||
module.exports = { | ||
MinHeap, | ||
MaxHeap | ||
}; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
{ | ||
"name": "@datastructures-js/heap", | ||
"version": "1.0.0", | ||
"description": "Heap (min & max) implementation in javascript", | ||
"main": "index.js", | ||
"scripts": { | ||
"test": "grunt test" | ||
}, | ||
"repository": { | ||
"type": "git", | ||
"url": "git+https://github.com/datastructures-js/heap.git" | ||
}, | ||
"keywords": [ | ||
"heap", | ||
"min heap", | ||
"max heap", | ||
"heap js", | ||
"heap es6", | ||
"heap sort", | ||
"heapify" | ||
], | ||
"author": "Eyas Ranjous <https://github.com/eyas-ranjous>", | ||
"license": "MIT", | ||
"bugs": { | ||
"url": "https://github.com/datastructures-js/heap/issues" | ||
}, | ||
"homepage": "https://github.com/datastructures-js/heap#readme", | ||
"devDependencies": { | ||
"chai": "^4.2.0", | ||
"eslint": "^6.7.2", | ||
"eslint-config-airbnb-base": "^14.0.0", | ||
"eslint-plugin-import": "^2.19.1", | ||
"grunt": "^1.0.4", | ||
"grunt-eslint": "^22.0.0", | ||
"grunt-mocha-istanbul": "^5.0.2", | ||
"grunt-mocha-test": "^0.13.3", | ||
"istanbul": "^0.4.5", | ||
"mocha": "^6.2.2" | ||
} | ||
} |
Oops, something went wrong.