forked from bitcoin/bitcoin
-
Notifications
You must be signed in to change notification settings - Fork 1.2k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge bitcoin#12830: [qt] [tests] Clarify address book error messages…
…, add tests 5109fc4 [tests] [qt] Add tests for address book manipulation via EditAddressDialog (James O'Beirne) 9c01be1 [tests] [qt] Introduce qt/test/util with a generalized ConfirmMessage (James O'Beirne) 8cdcaee [qt] Display more helpful message when adding a send address has failed (James O'Beirne) c5b2770 Add purpose arg to Wallet::getAddress (James O'Beirne) Pull request description: Addresses bitcoin#12796. When a user attempts to add to the address book a sending address which is already present as a receiving address, they're presented with a confusing error indicating the address is already present in the book, despite the fact that this row is currently invisible. 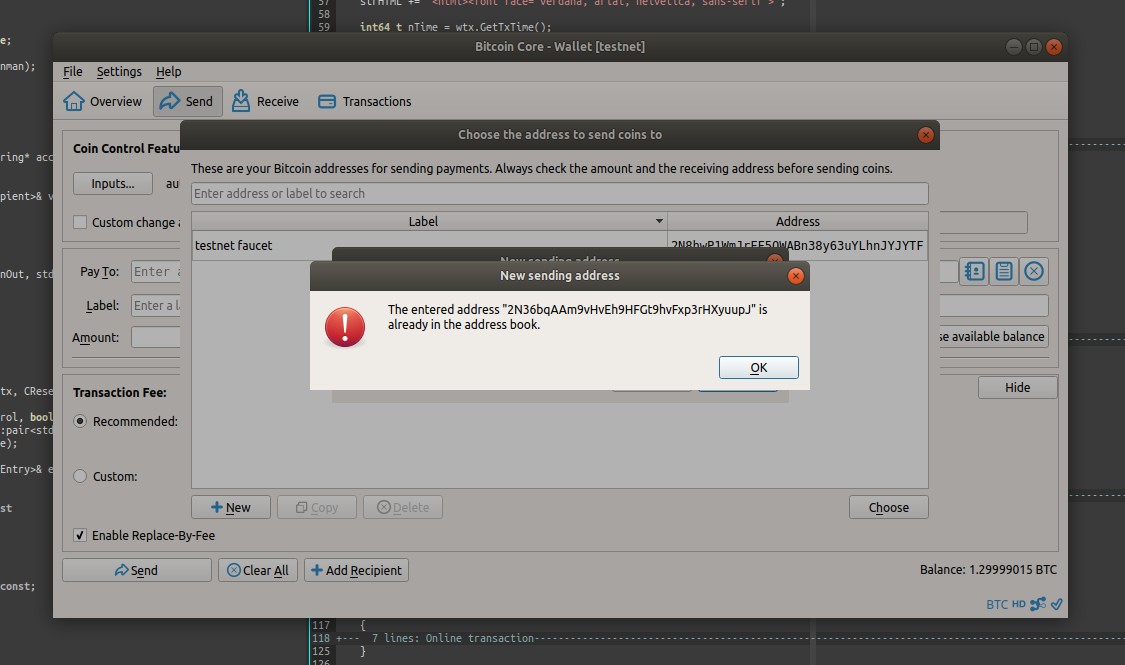 This change adds a more specific error message indicating its existence as a receiving address (as discussed in the linked issue). 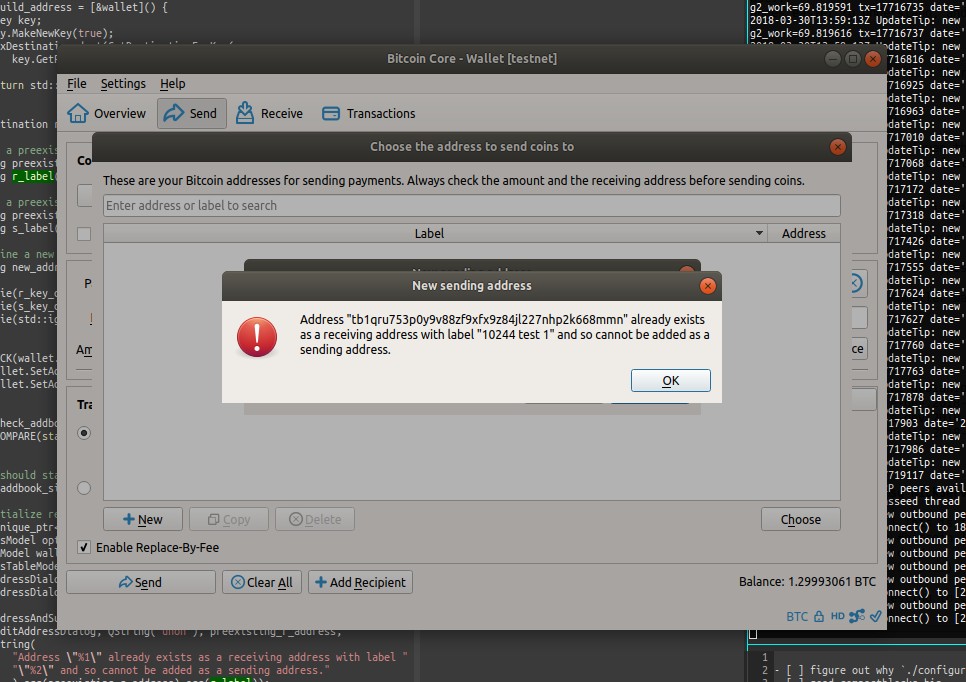 This change also adds some tests exercising use of the address book via QT. Adding so much test code for such a trivial change may seem weird, but it's my hope that this will make further test-writing for address book usage (and other QT features) more approachable. Tree-SHA512: fbdd5564f7a9a2380bbe437f3378e8d4d5fd9201efff4879b72bc23f2cc1c2eecaf2b811994c25070ee052422e48e47901787c2e62cc584774a997fe6a2a327a
- Loading branch information
Showing
18 changed files
with
286 additions
and
44 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,140 @@ | ||
#include <qt/test/addressbooktests.h> | ||
#include <qt/test/util.h> | ||
#include <test/test_dash.h> | ||
|
||
#include <interfaces/node.h> | ||
#include <qt/addressbookpage.h> | ||
#include <qt/addresstablemodel.h> | ||
#include <qt/editaddressdialog.h> | ||
#include <qt/callback.h> | ||
#include <qt/optionsmodel.h> | ||
#include <qt/qvalidatedlineedit.h> | ||
#include <qt/walletmodel.h> | ||
|
||
#include <key.h> | ||
#include <pubkey.h> | ||
#include <key_io.h> | ||
#include <wallet/wallet.h> | ||
|
||
#include <QTimer> | ||
#include <QMessageBox> | ||
|
||
namespace | ||
{ | ||
|
||
/** | ||
* Fill the edit address dialog box with data, submit it, and ensure that | ||
* the resulting message meets expectations. | ||
*/ | ||
void EditAddressAndSubmit( | ||
EditAddressDialog* dialog, | ||
const QString& label, const QString& address, QString expected_msg) | ||
{ | ||
QString warning_text; | ||
|
||
dialog->findChild<QLineEdit*>("labelEdit")->setText(label); | ||
dialog->findChild<QValidatedLineEdit*>("addressEdit")->setText(address); | ||
|
||
ConfirmMessage(&warning_text, 5); | ||
dialog->accept(); | ||
QCOMPARE(warning_text, expected_msg); | ||
} | ||
|
||
/** | ||
* Test adding various send addresses to the address book. | ||
* | ||
* There are three cases tested: | ||
* | ||
* - new_address: a new address which should add as a send address successfully. | ||
* - existing_s_address: an existing sending address which won't add successfully. | ||
* - existing_r_address: an existing receiving address which won't add successfully. | ||
* | ||
* In each case, verify the resulting state of the address book and optionally | ||
* the warning message presented to the user. | ||
*/ | ||
void TestAddAddressesToSendBook() | ||
{ | ||
TestChain100Setup test; | ||
std::shared_ptr<CWallet> wallet = std::make_shared<CWallet>(WalletLocation(), WalletDatabase::CreateMock()); | ||
bool firstRun; | ||
wallet->LoadWallet(firstRun); | ||
|
||
auto build_address = [wallet]() { | ||
CKey key; | ||
key.MakeNewKey(true); | ||
CTxDestination dest = key.GetPubKey().GetID(); | ||
|
||
return std::make_pair(dest, QString::fromStdString(EncodeDestination(dest))); | ||
}; | ||
|
||
CTxDestination r_key_dest, s_key_dest; | ||
|
||
// Add a preexisting "receive" entry in the address book. | ||
QString preexisting_r_address; | ||
QString r_label("already here (r)"); | ||
|
||
// Add a preexisting "send" entry in the address book. | ||
QString preexisting_s_address; | ||
QString s_label("already here (s)"); | ||
|
||
// Define a new address (which should add to the address book successfully). | ||
QString new_address; | ||
|
||
std::tie(r_key_dest, preexisting_r_address) = build_address(); | ||
std::tie(s_key_dest, preexisting_s_address) = build_address(); | ||
std::tie(std::ignore, new_address) = build_address(); | ||
|
||
{ | ||
LOCK(wallet->cs_wallet); | ||
wallet->SetAddressBook(r_key_dest, r_label.toStdString(), "receive"); | ||
wallet->SetAddressBook(s_key_dest, s_label.toStdString(), "send"); | ||
} | ||
|
||
auto check_addbook_size = [wallet](int expected_size) { | ||
QCOMPARE(static_cast<int>(wallet->mapAddressBook.size()), expected_size); | ||
}; | ||
|
||
// We should start with the two addresses we added earlier and nothing else. | ||
check_addbook_size(2); | ||
|
||
// Initialize relevant QT models. | ||
auto node = interfaces::MakeNode(); | ||
OptionsModel optionsModel(*node); | ||
AddWallet(wallet); | ||
WalletModel walletModel(std::move(node->getWallets()[0]), *node, &optionsModel); | ||
RemoveWallet(wallet); | ||
EditAddressDialog editAddressDialog(EditAddressDialog::NewSendingAddress); | ||
editAddressDialog.setModel(walletModel.getAddressTableModel()); | ||
|
||
EditAddressAndSubmit( | ||
&editAddressDialog, QString("uhoh"), preexisting_r_address, | ||
QString( | ||
"Address \"%1\" already exists as a receiving address with label " | ||
"\"%2\" and so cannot be added as a sending address." | ||
).arg(preexisting_r_address).arg(r_label)); | ||
|
||
check_addbook_size(2); | ||
|
||
EditAddressAndSubmit( | ||
&editAddressDialog, QString("uhoh, different"), preexisting_s_address, | ||
QString( | ||
"The entered address \"%1\" is already in the address book with " | ||
"label \"%2\"." | ||
).arg(preexisting_s_address).arg(s_label)); | ||
|
||
check_addbook_size(2); | ||
|
||
// Submit a new address which should add successfully - we expect the | ||
// warning message to be blank. | ||
EditAddressAndSubmit( | ||
&editAddressDialog, QString("new"), new_address, QString("")); | ||
|
||
check_addbook_size(3); | ||
} | ||
|
||
} // namespace | ||
|
||
void AddressBookTests::addressBookTests() | ||
{ | ||
TestAddAddressesToSendBook(); | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,15 @@ | ||
#ifndef BITCOIN_QT_TEST_ADDRESSBOOKTESTS_H | ||
#define BITCOIN_QT_TEST_ADDRESSBOOKTESTS_H | ||
|
||
#include <QObject> | ||
#include <QTest> | ||
|
||
class AddressBookTests : public QObject | ||
{ | ||
Q_OBJECT | ||
|
||
private Q_SLOTS: | ||
void addressBookTests(); | ||
}; | ||
|
||
#endif // BITCOIN_QT_TEST_ADDRESSBOOKTESTS_H |
Oops, something went wrong.