-
Notifications
You must be signed in to change notification settings - Fork 287
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge pull request #1114 from Tanaya555/main
Add files via upload
- Loading branch information
Showing
33 changed files
with
9,073 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,14 @@ | ||
# Articooz 🖊📝 | ||
## A platform to post blogs and articles, follow each other, and even have conversations. | ||
|
||
## ➡ [Live Site](https://articooz.herokuapp.com/) ⬅ | ||
--- | ||
## Screenshots | ||
|
||
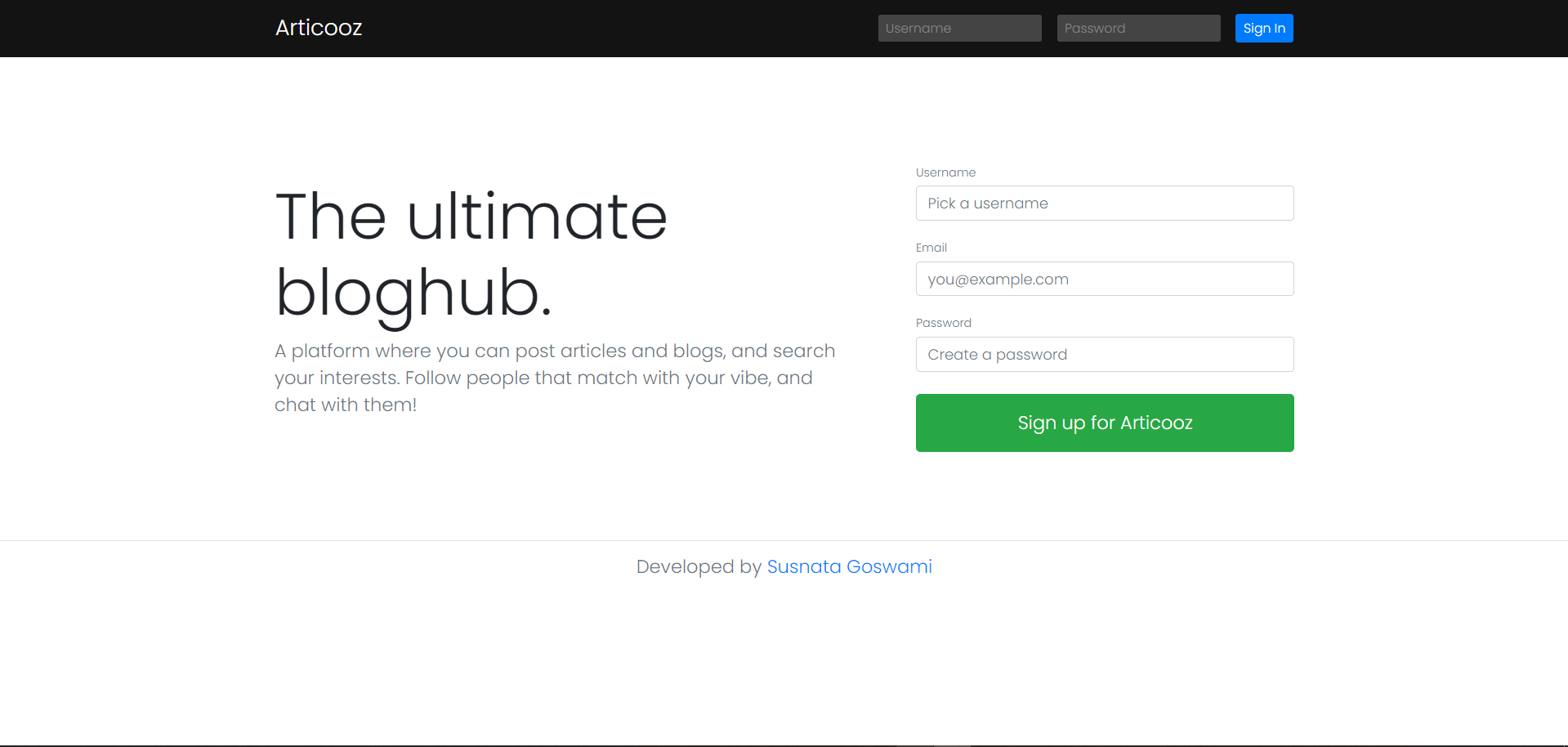 | ||
|
||
|
||
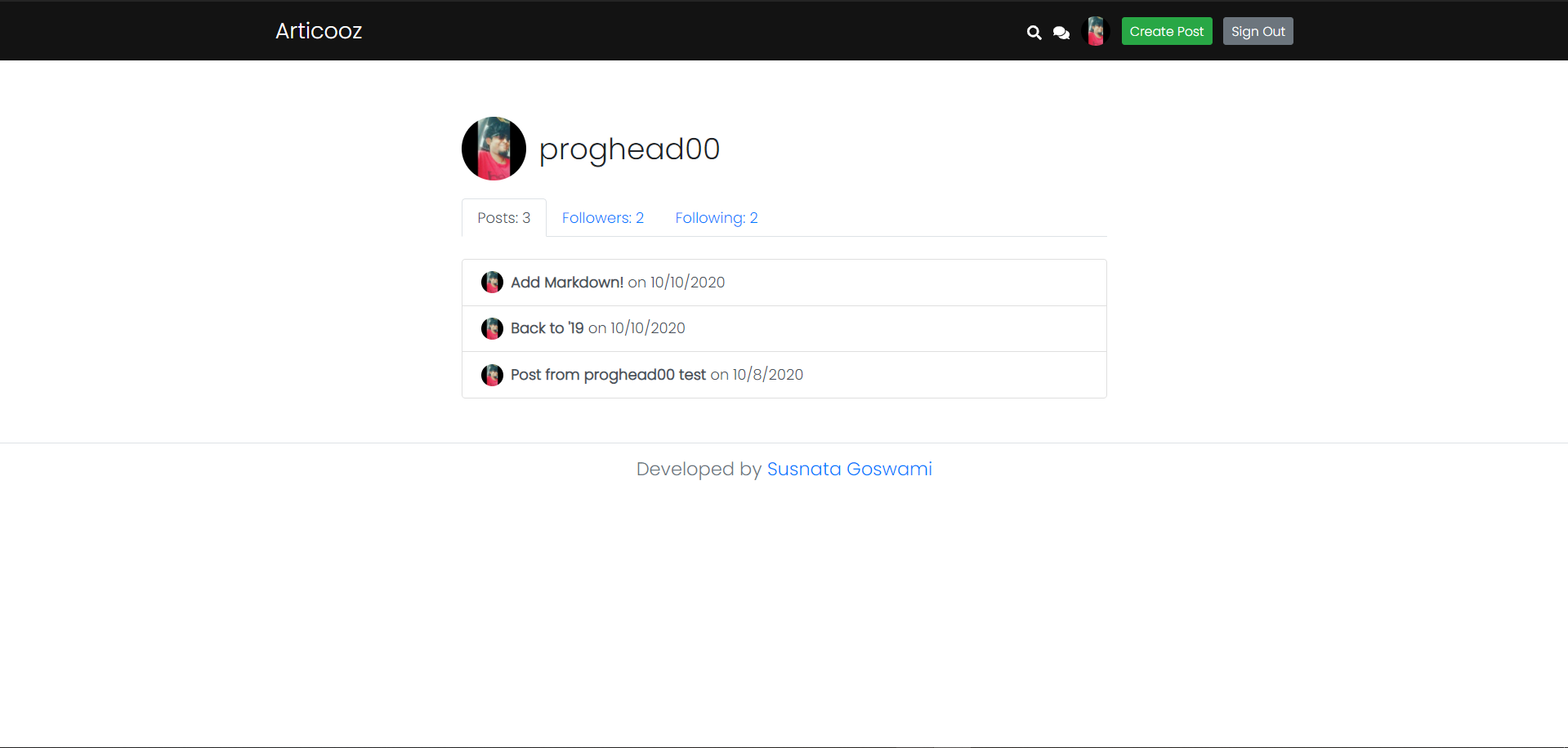 | ||
|
||
|
||
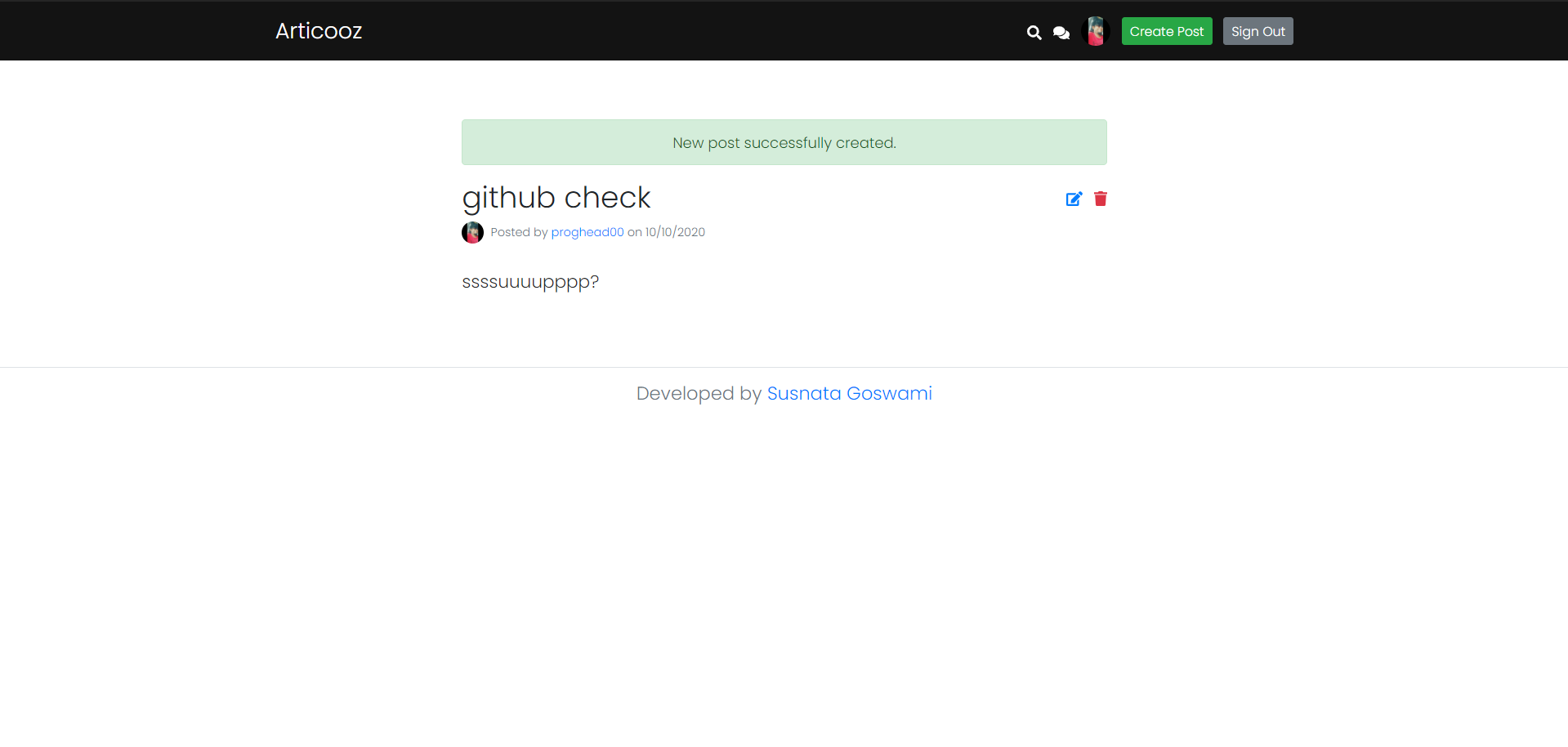 |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,119 @@ | ||
const express = require("express"); | ||
const session = require("express-session"); | ||
const MongoStore = require("connect-mongo")(session); | ||
const flash = require("connect-flash"); | ||
const markdown = require("marked"); | ||
const csrf = require("csurf"); | ||
const app = express(); | ||
const sanitizeHTML = require("sanitize-html"); | ||
|
||
app.use(express.urlencoded({ extended: false })); | ||
app.use(express.json()); | ||
|
||
app.use("/api", require("./router-api")); | ||
|
||
let sessionOptions = session({ | ||
secret: "JavaScript is sooooooooo coool", | ||
store: new MongoStore({ client: require("./db") }), | ||
resave: false, | ||
saveUninitialized: false, | ||
cookie: { maxAge: 1000 * 60 * 60 * 24, httpOnly: true }, | ||
}); | ||
|
||
app.use(sessionOptions); | ||
app.use(flash()); | ||
|
||
app.use(function (req, res, next) { | ||
// make our markdown function available from within ejs templates | ||
res.locals.filterUserHTML = function (content) { | ||
return sanitizeHTML(markdown(content), { | ||
allowedTags: [ | ||
"p", | ||
"br", | ||
"ul", | ||
"ol", | ||
"li", | ||
"strong", | ||
"bold", | ||
"i", | ||
"em", | ||
"h1", | ||
"h2", | ||
"h3", | ||
"h4", | ||
"h5", | ||
"h6", | ||
], | ||
allowedAttributes: {}, | ||
}); | ||
}; | ||
|
||
// make all error and success flash messages available from all templates | ||
res.locals.errors = req.flash("errors"); | ||
res.locals.success = req.flash("success"); | ||
|
||
// make current user id available on the req object | ||
if (req.session.user) { | ||
req.visitorId = req.session.user._id; | ||
} else { | ||
req.visitorId = 0; | ||
} | ||
|
||
// make user session data available from within view templates | ||
res.locals.user = req.session.user; | ||
next(); | ||
}); | ||
|
||
const router = require("./router"); | ||
|
||
app.use(express.static("public")); | ||
app.set("views", "views"); | ||
app.set("view engine", "ejs"); | ||
|
||
app.use(csrf()); | ||
|
||
app.use(function (req, res, next) { | ||
res.locals.csrfToken = req.csrfToken(); | ||
next(); | ||
}); | ||
|
||
app.use("/", router); | ||
|
||
app.use(function (err, req, res, next) { | ||
if (err) { | ||
if (err.code == "EBADCSRFTOKEN") { | ||
req.flash("errors", "Cross site request forgery detected."); | ||
req.session.save(() => res.redirect("/")); | ||
} else { | ||
res.render("404"); | ||
} | ||
} | ||
}); | ||
|
||
const server = require("http").createServer(app); | ||
const io = require("socket.io")(server); | ||
|
||
io.use(function (socket, next) { | ||
sessionOptions(socket.request, socket.request.res, next); | ||
}); | ||
|
||
io.on("connection", function (socket) { | ||
if (socket.request.session.user) { | ||
let user = socket.request.session.user; | ||
|
||
socket.emit("welcome", { username: user.username, avatar: user.avatar }); | ||
|
||
socket.on("chatMessageFromBrowser", function (data) { | ||
socket.broadcast.emit("chatMessageFromServer", { | ||
message: sanitizeHTML(data.message, { | ||
allowedTags: [], | ||
allowedAttributes: {}, | ||
}), | ||
username: user.username, | ||
avatar: user.avatar, | ||
}); | ||
}); | ||
} | ||
}); | ||
|
||
module.exports = server; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,36 @@ | ||
const Follow = require("../models/Follow"); | ||
|
||
exports.addFollow = function (req, res) { | ||
let follow = new Follow(req.params.username, req.visitorId); | ||
follow | ||
.create() | ||
.then(() => { | ||
req.flash("success", `Successfully followed ${req.params.username}`); | ||
req.session.save(() => res.redirect(`/profile/${req.params.username}`)); | ||
}) | ||
.catch((errors) => { | ||
errors.forEach((error) => { | ||
req.flash("errors", error); | ||
}); | ||
req.session.save(() => res.redirect("/")); | ||
}); | ||
}; | ||
|
||
exports.removeFollow = function (req, res) { | ||
let follow = new Follow(req.params.username, req.visitorId); | ||
follow | ||
.delete() | ||
.then(() => { | ||
req.flash( | ||
"success", | ||
`Successfully stopped following ${req.params.username}` | ||
); | ||
req.session.save(() => res.redirect(`/profile/${req.params.username}`)); | ||
}) | ||
.catch((errors) => { | ||
errors.forEach((error) => { | ||
req.flash("errors", error); | ||
}); | ||
req.session.save(() => res.redirect("/")); | ||
}); | ||
}; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,98 @@ | ||
const Post = require("../models/Post"); | ||
|
||
exports.viewCreateScreen = function (req, res) { | ||
res.render("create-post"); | ||
}; | ||
|
||
exports.create = function (req, res) { | ||
let post = new Post(req.body, req.session.user._id); | ||
post | ||
.create() | ||
.then(function (newId) { | ||
req.flash("success", "New post successfully created."); | ||
req.session.save(() => res.redirect(`/post/${newId}`)); | ||
}) | ||
.catch(function (errors) { | ||
errors.forEach((error) => req.flash("errors", error)); | ||
req.session.save(() => res.redirect("/create-post")); | ||
}); | ||
}; | ||
|
||
exports.viewSingle = async function (req, res) { | ||
try { | ||
let post = await Post.findSingleById(req.params.id, req.visitorId); | ||
res.render("single-post-screen", { post: post, title: post.title }); | ||
} catch { | ||
res.render("404"); | ||
} | ||
}; | ||
|
||
exports.viewEditScreen = async function (req, res) { | ||
try { | ||
let post = await Post.findSingleById(req.params.id, req.visitorId); | ||
if (post.isVisitorOwner) { | ||
res.render("edit-post", { post: post }); | ||
} else { | ||
req.flash("errors", "You do not have permission to perform that action."); | ||
req.session.save(() => res.redirect("/")); | ||
} | ||
} catch { | ||
res.render("404"); | ||
} | ||
}; | ||
|
||
exports.edit = function (req, res) { | ||
let post = new Post(req.body, req.visitorId, req.params.id); | ||
post | ||
.update() | ||
.then((status) => { | ||
// the post was successfully updated in the database | ||
// or user did have permission, but there were validation errors | ||
if (status == "success") { | ||
// post was updated in db | ||
req.flash("success", "Post successfully updated."); | ||
req.session.save(function () { | ||
res.redirect(`/post/${req.params.id}/edit`); | ||
}); | ||
} else { | ||
post.errors.forEach(function (error) { | ||
req.flash("errors", error); | ||
}); | ||
req.session.save(function () { | ||
res.redirect(`/post/${req.params.id}/edit`); | ||
}); | ||
} | ||
}) | ||
.catch(() => { | ||
// a post with the requested id doesn't exist | ||
// or if the current visitor is not the owner of the requested post | ||
req.flash("errors", "You do not have permission to perform that action."); | ||
req.session.save(function () { | ||
res.redirect("/"); | ||
}); | ||
}); | ||
}; | ||
|
||
exports.delete = function (req, res) { | ||
Post.delete(req.params.id, req.visitorId) | ||
.then(() => { | ||
req.flash("success", "Post successfully deleted."); | ||
req.session.save(() => | ||
res.redirect(`/profile/${req.session.user.username}`) | ||
); | ||
}) | ||
.catch(() => { | ||
req.flash("errors", "You do not have permission to perform that action."); | ||
req.session.save(() => res.redirect("/")); | ||
}); | ||
}; | ||
|
||
exports.search = function (req, res) { | ||
Post.search(req.body.searchTerm) | ||
.then((posts) => { | ||
res.json(posts); | ||
}) | ||
.catch(() => { | ||
res.json([]); | ||
}); | ||
}; |
Oops, something went wrong.