-
Notifications
You must be signed in to change notification settings - Fork 0
devlog#01 Nuxt3 server routes
If you consider VueJs as intuitive, have a look at Nuxt 3 ! While you can benefit from server side rendering, a lot of features have been added to simplfy your development process.
I once used the 2nd version of Nuxt for my uni student council's website and it was great. However, I can confirm that new version of Nuxt is a game changer.
The most amazing feature for me is the server routes. It allows creating an API using nuxt's routing features, which allows you to avoid managing a second server for your back-end. Perfect if you have a not-so-big monolithic project.
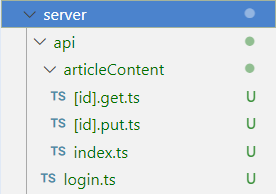
- Nuxt automatically scans files inside the
@/server/api
directory. - Each files will be registered automatically as routes. Typescript
.ts
is supported out of the box. - To add a request parameter, surround your
[parameter]
with brackets. - Suffix your file with
.get
,.post
... to match request's HTTP Method. Pretty intuitive.
I cannot explain all the features of automatic routing in this log, so have a look at Nuxt3's documentation.
Code | Result |
---|---|
/**
* This route just shows hello
* @file /server/api/articleContent/index.ts
*/
export default defineHandler((event: IncomingMessage) => {
return "Hello world!";
}); |
GET /api/articleContent/ |
Code | Result |
---|---|
import { articleExists, saveArticle } from "@/server/helpers/articles";
/**
* This route updates an article
* @file articleContent/[id].put.ts
*/
export default defineHandler(async (event: IncomingMessage) => {
// get request parameters with event.context.params
const articleId = event.context.params.id;
// get request body as JS object with useBody (auto-imported)
const body = await useBody(event);
// return HTTP errors by throwing createError(statusCode, statusMessage)
if (!articleExists(articleId)) {
throw createError({ statusCode: 404, statusMessage: `Article ${articleId} not found` });
}
await saveArticle(body);
// you can directly a JS object that will be serialized.
return {
status: "success",
message: `Updated article ${articleId}`,
};
}); |
PUT /api/articleContent/1 (where 1 is an existing article) PUT /api/articleContent/99 (where 99 is an article that does not exists) |