-
-
Notifications
You must be signed in to change notification settings - Fork 5
Use with Unity
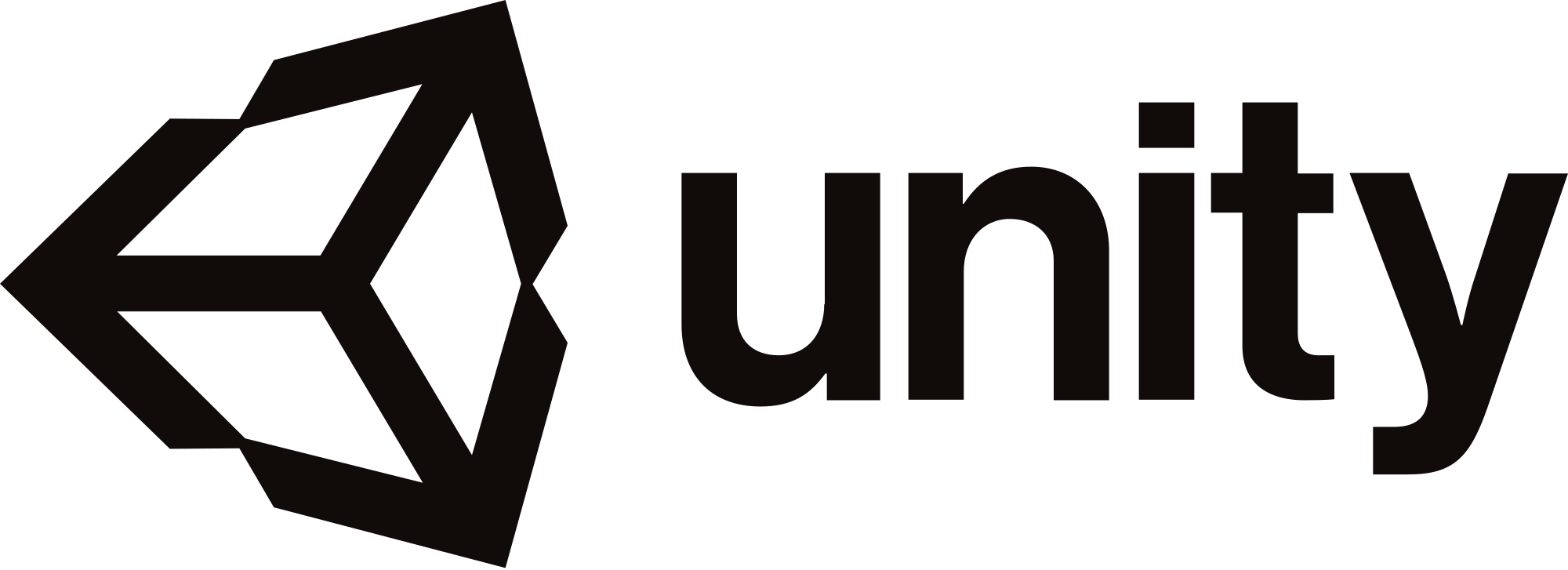
;tldr; look at this example: https://github.com/JensKrumsieck/ChemSharpUnityExample
ChemSharp does support the Unity Game Engine for Visualization of e.g. Molecules. You simply need to use it in a MonoBehaviour. The example shown below creates a sphere with correct size wherever an atom is located and adds a basic material.
using System;
using ChemSharp.Molecules;
using UnityEngine;
public class MoleculeTest : MonoBehaviour
{
public string path = @"Assets/CuHETMP.cif";
void Start()
{
var mol = Molecule.FromFile(path);
foreach (var atom in mol.Atoms)
{
var obj = GameObject.CreatePrimitive(PrimitiveType.Sphere);
obj.transform.position = new Vector3(atom.Location.X, atom.Location.Y, atom.Location.Z);
obj.transform.localScale =
new Vector3(atom.CovalentRadius, atom.CovalentRadius, atom.CovalentRadius) / 100f;
var mat = new Material(Shader.Find("Standard"));
ColorUtility.TryParseHtmlString(atom.Color, out var col);
mat.color = col;
obj.GetComponent<Renderer>().material = mat;
}
foreach (var bond in mol.Bonds)
{
var obj = GameObject.CreatePrimitive(PrimitiveType.Cylinder);
var start = new Vector3(bond.Atom1.Location.X, bond.Atom1.Location.Y, bond.Atom1.Location.Z);
var end = new Vector3(bond.Atom2.Location.X, bond.Atom2.Location.Y, bond.Atom2.Location.Z);
var loc = Vector3.Lerp(start, end, 0.5f);
var lineVector = end - start;
var axis = Vector3.Cross(Vector3.up, lineVector.normalized);
var rad = Mathf.Acos(Vector3.Dot(Vector3.up, lineVector.normalized));
var matrix = Quaternion.AxisAngle(axis, rad);
obj.transform.localScale = new Vector3(0.05f, lineVector.magnitude / 2f, 0.05f);
obj.transform.position = loc;
obj.transform.rotation = matrix;
}
}
}
You need to bring in the Dependencies yourself. The easiest way to bring in NuGet Packages into Unity is to create a separate C# Project (somewhere on your device) and reference all your needed NuGet Packages in this project. However the Project has to target the same Framework as Unity (e.g. netstandard2.1).
Add a nuget.config file in the solutions root directory:
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<config>
<add key="globalPackagesFolder" value="packages"/>
</config>
<packageRestore>
<add key="enabled" value="true" />
<add key="automatic" value="true" />
</packageRestore>
</configuration>
and use this PowerShell Script to restore dependencies and copy the needed dll-Files into a "deps" Folder. Unity will do the rest for you if your destination folder is located in your project folder...
$source=$MyInvocation.MyCommand.Path
$path=Split-Path $source -Parent
$dest=$path+"..\..\ChemSharpUnity\Assets\packages"
$source=$path+"\packages\"
dotnet restore
New-Item $dest -ItemType Directory -Force
$filter = [regex] "lib\\netstandard2.0"
Get-ChildItem -Path $source -Recurse -Include *.dll | Where-Object {$_.FullName -match $filter} | Copy-Item -Destination $dest
A Example csproj-File can look like the following:
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>netstandard2.1</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="ChemSharp.Molecules" Version="2.0.1" />
</ItemGroup>
</Project>