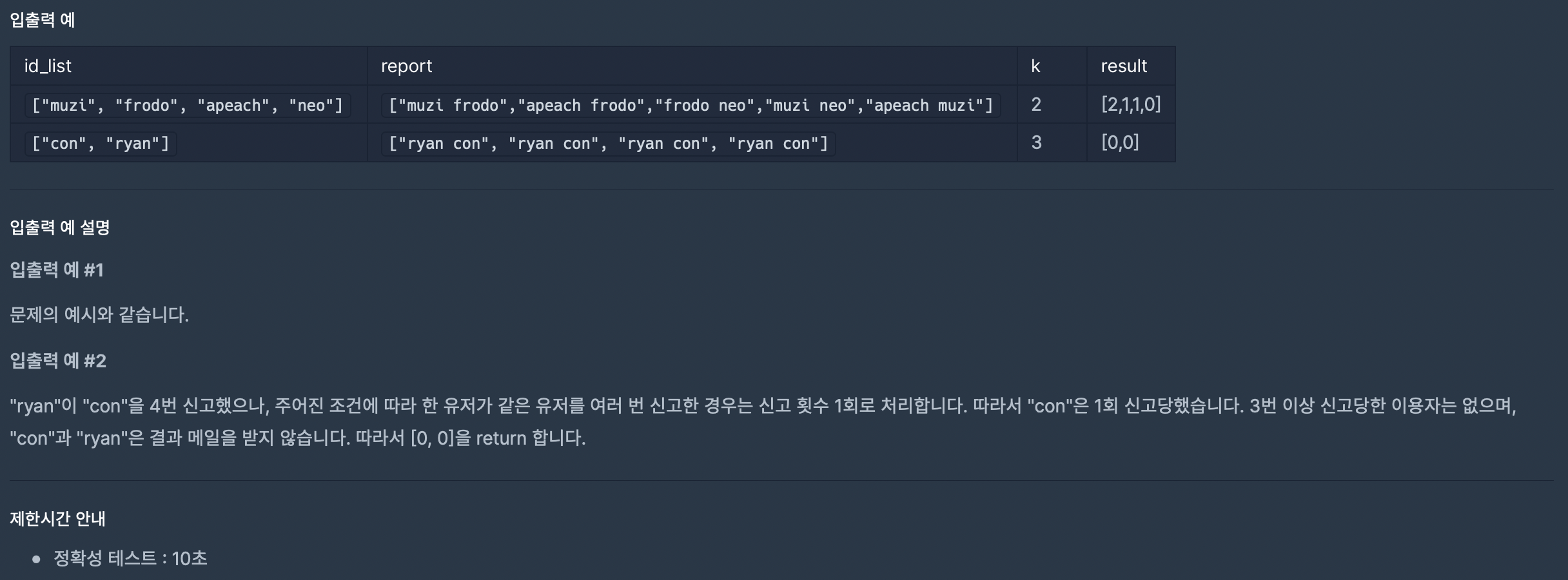
import Foundation
func solution(_ id_list:[String], _ report:[String], _ k:Int) -> [Int] {
var answer = [Int](repeating: 0, count: id_list.count)
// list of people who reported key
var reported: [String: [String]] = [:]
// 0: reported, 1: was reported
var detail: [String]
// index
var id_index: [String: Int] = [:]
for i in 0..<id_list.count {
id_index[id_list[i]] = i
}
// init reported
for rpt in report {
detail = rpt.split(separator: " ").map{ String($0) }
if !reported.keys.contains(detail[1]) {
reported[detail[1]] = [detail[0]]
} else if !reported[detail[1]]!.contains(detail[0]) {
reported[detail[1]]!.append(detail[0])
}
}
// count of mail to receive
for key in reported.filter({ $0.value.count >= k}) {
for name in key.value {
answer[id_index[name]!] += 1
}
}
return answer
}
import java.util.*;
class Solution {
public int[] solution(String[] id_list, String[] report, int k) {
int[] answer = new int[id_list.length];
HashMap<String, HashSet<String>> map = new HashMap<>();
for(String item : report){
String key = item.split(" ")[1];
String value = item.split(" ")[0];
if(!map.keySet().contains(key)){
map.put(key, new HashSet<>(Arrays.asList(value)));
}
else{
map.get(key).add(value);
}
}
for(String key : map.keySet()){
if(k <= map.get(key).size()){
for(int i = 0; i < id_list.length; i++){
if(map.get(key).contains(id_list[i])){
answer[i]++;
}
}
}
}
return answer;
}
}
import java.util.*;
class Solution {
public int[] solution(String[] id_list, String[] report, int k) {
StringTokenizer st;
int size = id_list.length;
int[] answer = new int[size];
Map<String, HashSet<String>> map = new HashMap<>();
Map<String, Integer> id = new HashMap<>();
for(int i=0; i<size; i++) {
map.put(id_list[i], new HashSet<>());
id.put(id_list[i], i);
}
for(String r:report) {
st = new StringTokenizer(r);
String from = st.nextToken();
String to = st.nextToken();
map.get(to).add(from);
}
for(int i=0; i<size; i++) {
HashSet<String> set = map.get(id_list[i]);
int length = set.size();
if(length < k) continue;
for(String from:set) {
answer[id.get(from)]++;
}
}
return answer;
}
}
import java.util.*;
class Solution {
public int[] solution(String[] id_list, String[] report_list, int k) {
Map<String, HashSet<String>> reportHashMap = new HashMap<>();
Map<String, Integer> resultHashMap = new LinkedHashMap<>();
for (String id : id_list) {
resultHashMap.put(id, 0);
reportHashMap.put(id, new HashSet<>());
}
for (String report : report_list) {
String[] reportInformation = report.split(" ");
reportHashMap.get(reportInformation[1]).add(reportInformation[0]);
}
for (String reported : reportHashMap.keySet()) {
if (reportHashMap.get(reported).size() >= k) {
for (String reporter : reportHashMap.get(reported)) {
resultHashMap.put(reporter, resultHashMap.get(reporter) + 1);
}
}
}
return resultHashMap.values().stream().mapToInt(i -> i).toArray();
}
}
import java.util.*;
class Solution {
public int[] solution(String[] id_list, String[] report, int k) {
int[] answer = new int[id_list.length];
Map<String, Integer> idx = new HashMap<>(); //유저 정보 인덱스 저장
Map<String, HashSet<String>> user = new HashMap<>(); //유저별 신고 정보 저장 -> 중복X
//신고 정보 저장을 위한 초기화
for(int i=0; i<id_list.length; i++){
idx.put(id_list[i], i);
user.put(id_list[i], new HashSet<>());
}
//신고 저장
//해당 유저가 몇번 신고당했는지에 따라서 결과 출력 -> 신고 당한 사람 입장에서 계산
for(int i=0; i<report.length; i++){
StringTokenizer st = new StringTokenizer(report[i]); //A(신고자) B(신고 당한 사람)
String from = st.nextToken();
String to = st.nextToken();
//한 신고자가 다른 동일한 사람에게 신고 불가능
user.get(to).add(from); //신고 당한 사람에게 신고 한 사람 저장 -> 한번만 저장됨(Set)
}
//신고 메일
for(int i=0; i<id_list.length; i++){
HashSet<String> mail = user.get(id_list[i]);
//신고자가 보낸 메일 중 인정이 신고 당한 사람이 k번 넘겨서 메일이 보내진 횟수 출력
if(mail.size() >= k){
for(String name : mail){
answer[idx.get(name)]++;
}
}
}
return answer;
}
}