-
Notifications
You must be signed in to change notification settings - Fork 810
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Problem with selection of a date and then scroll to another month where i'm now able to select two dates. The first date doesn't get deselected #608
Comments
You did not implement the will display cell function. Can you let me know if this fixed your issue? |
Yes this solved my issue. Thank you! Have a nice day :) |
awesome. |
@cyrilzakka did you reset your cells. |
@patchthecode I seem to have missed this step, my apologies. Was it in one of the first two videos? Where can I find it? |
@cyrilzakka I sorry, i misread the question you asked. |
issue resolved. user was dequeueing inside the willDisplay function. |
Hello! Thank you for all the wonderful work!
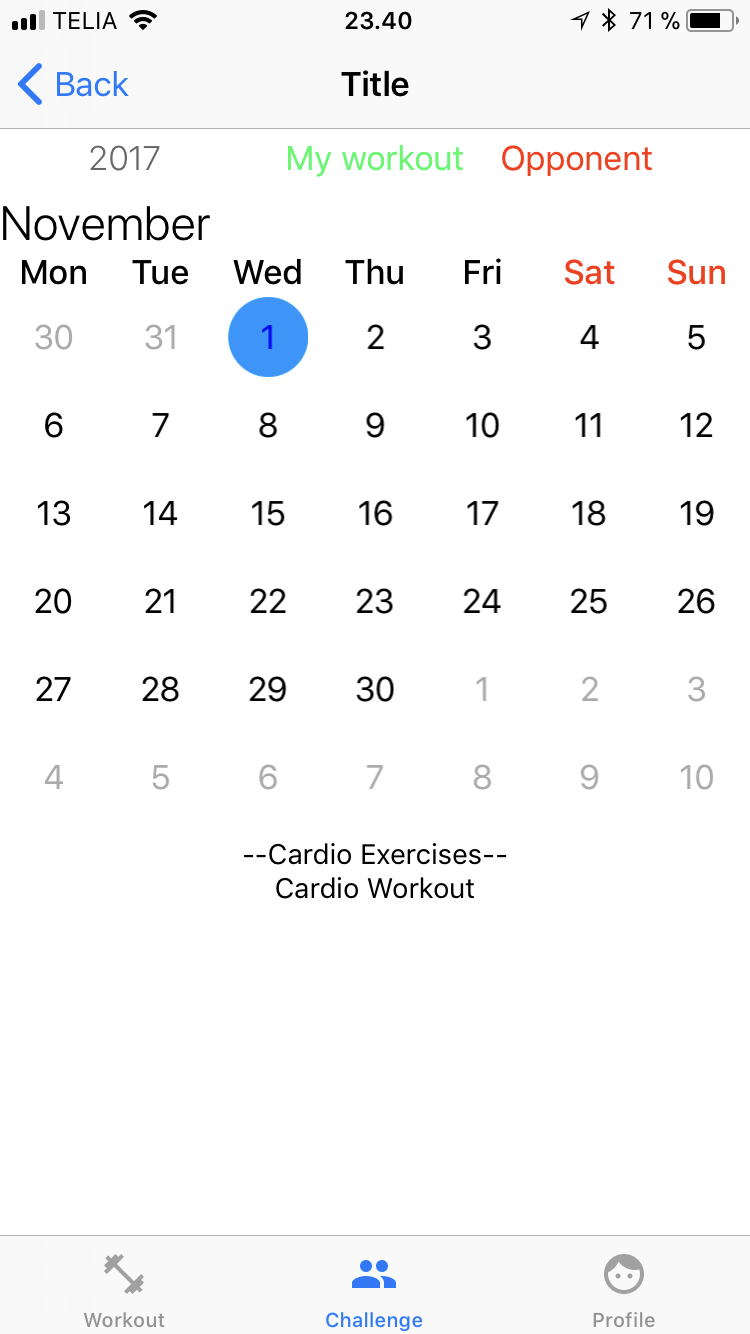
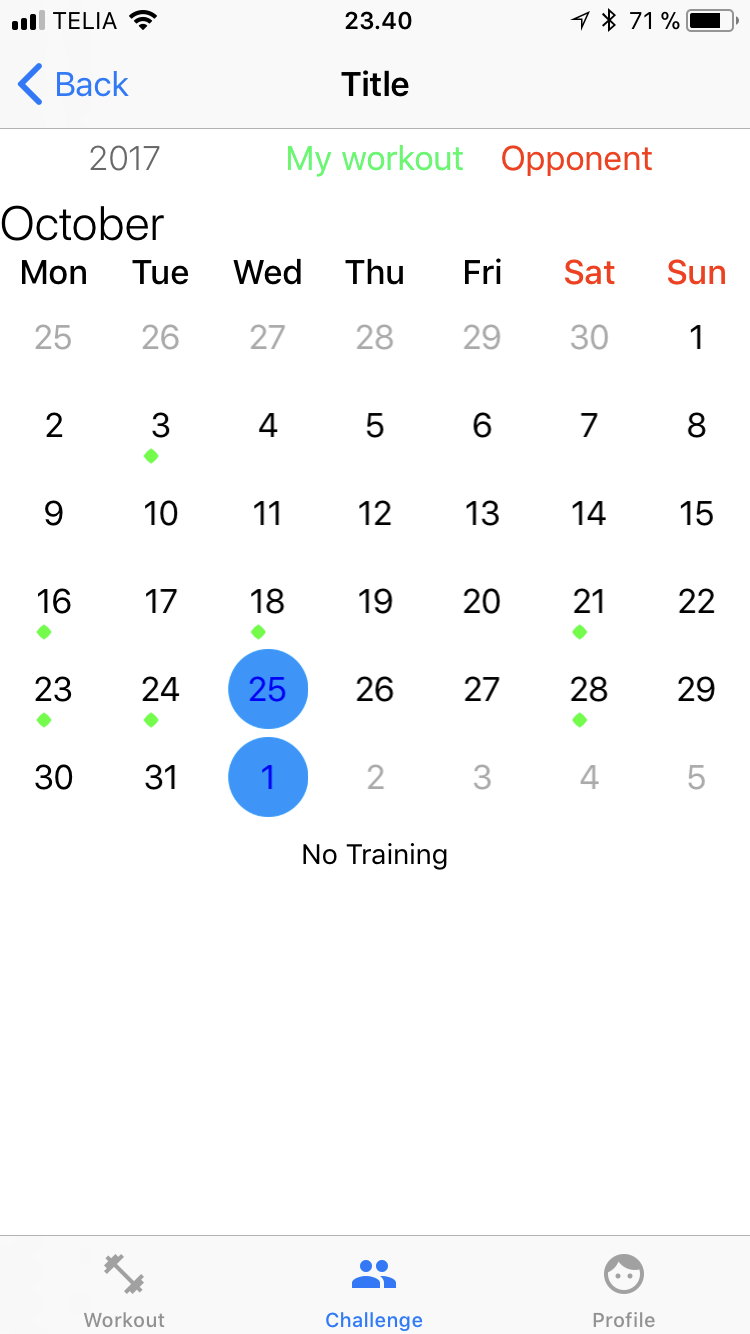
I'm using version 7.1.1
I have a problem with the selection of a date. If i select a date in a month and i fx scroll to the month before where this date is also shown, it won't be deselected. So now i'm marking 2 dates. I've attached two pictures to show it. My code is also attached.
Thank you!
The text was updated successfully, but these errors were encountered: