-
Notifications
You must be signed in to change notification settings - Fork 1.6k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Better type inference for decorators. #774
Comments
Pyright is doing the right thing in this case. It is inferring as much as it can given the information it is provided within your code plus the stdlib type stubs. The
Note that I don't know enough about functools or the wraps decorator method to say whether this is a bug in the type stub. Should it be using a TypeVar that is bound to an _AnyCallable? If you think this is a bug, please report it in the typeshed repo. In any case, there's a simple workaround that you can apply to your sample. Define a TypeVar that is bound to a function type, and use it in the declaration of
|
Thank you so much for your clear explanation and brilliant suggestion! That's exactly what I need. ❤️ |
If I do this:
I get the following error (at the
Did I do something wrong? Can this error be removed? |
In Python 3.9, there's not a great solution to this problem. The best workaround I can offer is the following: return cast(_TFunc, wrapper) In Python 3.10, there's a new facility called a Here's how it would look with the new capability: from typing import Callable, TypeVar
from typing_extensions import ParamSpec
_P = ParamSpec("_P")
_R = TypeVar("_R")
def decorator(f: Callable[_P, _R]) -> Callable[_P, _R]:
def wrapper(*args: _P.args, **kwargs: _P.kwargs):
print("wrapper", *args, **kwargs)
return f(*args, **kwargs)
return wrapper |
Thanks for the explanation and the hint to PEP 612! It's great to see how the language keeps evolving. |
Just side note here: support for PEP 612 in |
Is there a way to apply this for class decorators as well? I lose intellisense documentation and autosuggestions when I decorate a class. I'm in python 3.10.5 by the way. |
If you'd like help annotating your class decorator, please post a question to the discussions forum and include the unannotated code. |
I'm in python 3.12.2, I did similar modification on my class base decoreator like following
Pylance and mypy are happy, before this modification, Pylance is reporting "reportMissingParameterType" |
Is your feature request related to a problem? Please describe.
Decorated functions will lose all of its signatures if used with decorators. I know some decorators might change the signature of the original function, but most of them are just using
*args
and**kwargs
to proxy all the parameters.Please see this example:
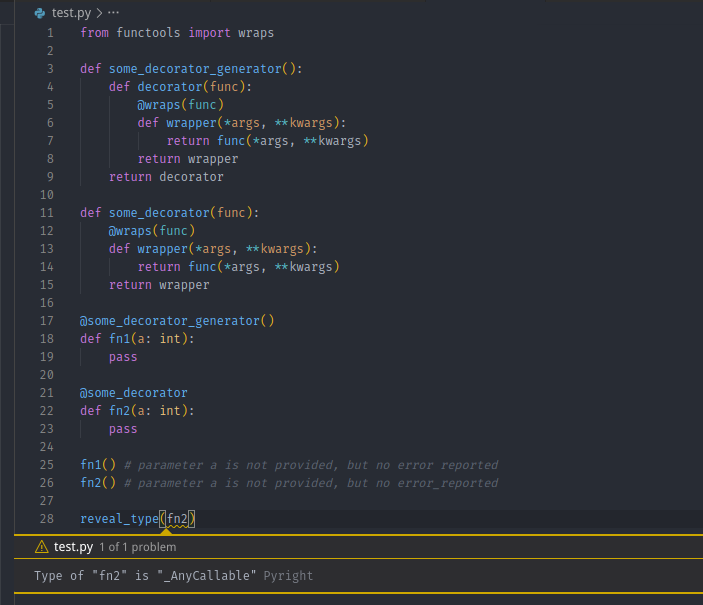
Describe the solution you'd like
Not sure if it's easy, but I hope a function decorated by decorators that only proxy all the parameters can keep its original signatures. this will make decorators a lot safer to use.
Thanks!
The text was updated successfully, but these errors were encountered: