Area chart is not refreshed when chart data is updated #15189
Labels
Fluent UI react (v8)
Issues about @fluentui/react (v8)
Needs: Investigation
The Shield Dev should investigate this issue and propose a fix
Package: charting
Environment Information
Please provide a reproduction of the bug in a codepen:
Area chart is not refreshed when chart data is updated
Code to repro it
Actual behavior:
Click the "Update data" button. The chart data is not updated.
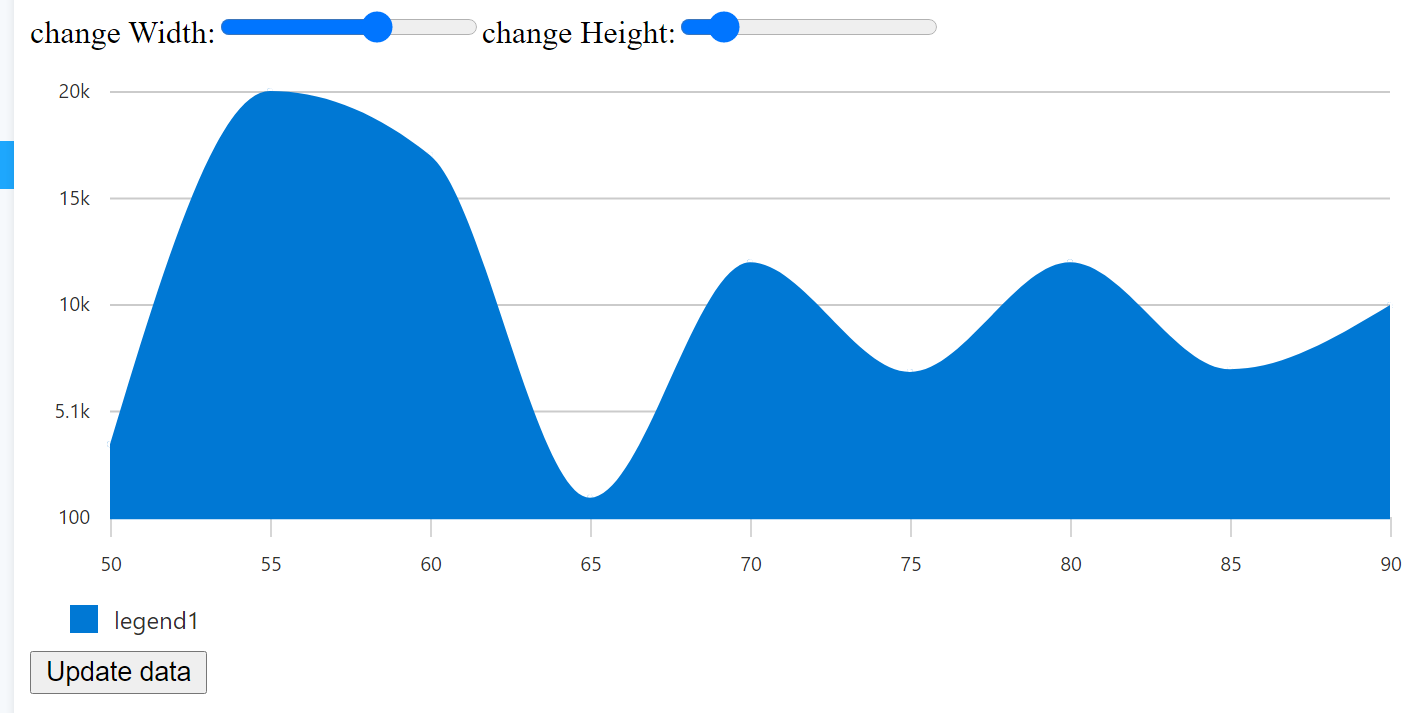
Expected behavior:
Click the "Update data" button. The chart is as below
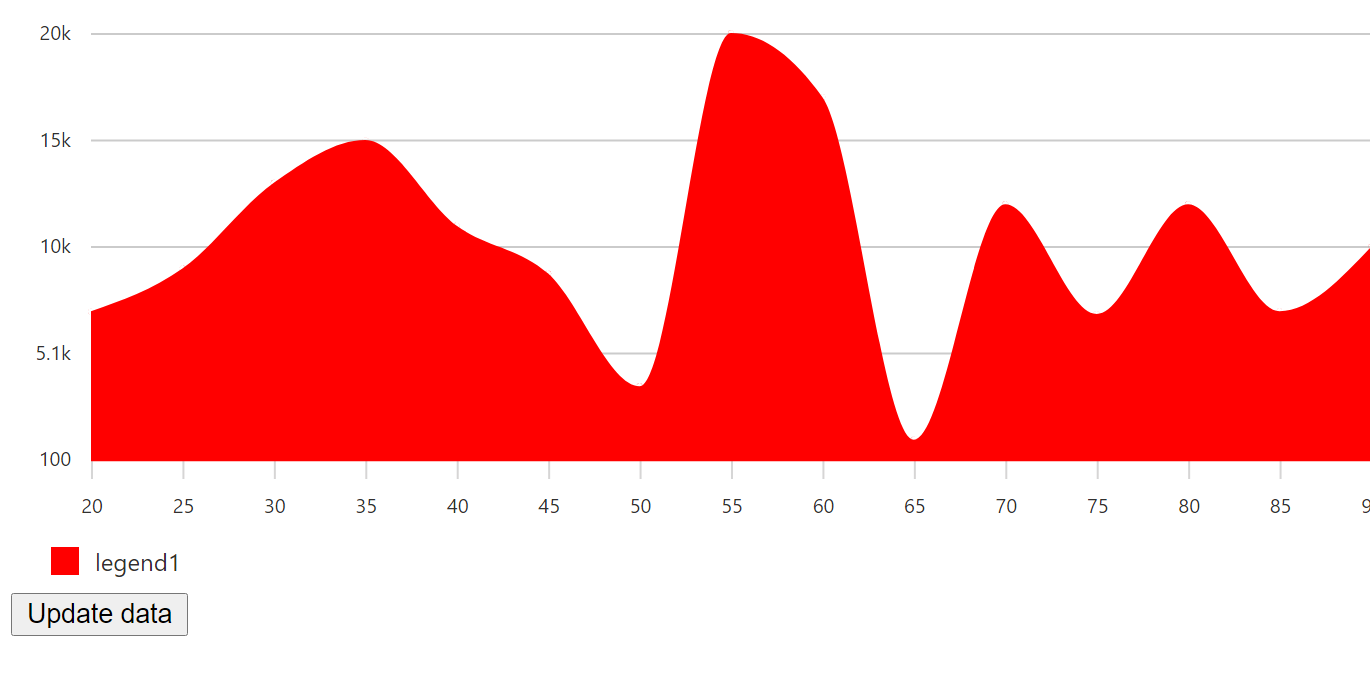
Priorities and help requested:
Are you willing to submit a PR to fix? (Yes, No)
Requested priority: (Blocking)
Products/sites affected: (if applicable)
The text was updated successfully, but these errors were encountered: