This repository has been archived by the owner on Jan 29, 2025. It is now read-only.
Inconsistency in matrix byte representation #1656
Labels
area: back-end
Outputs of shader conversion
kind: bug
Something isn't working
lang: Metal
Metal Shading Language
I am running the same program on Linux (Vulkan backend) and on macOS (Metal backend) and getting two different results, because I have a uniform containing a transformation matrix and it is not being translated the same way on both platforms.
In this example, the transformation matrix is meant to be applied to the image of the goat.
Linux:
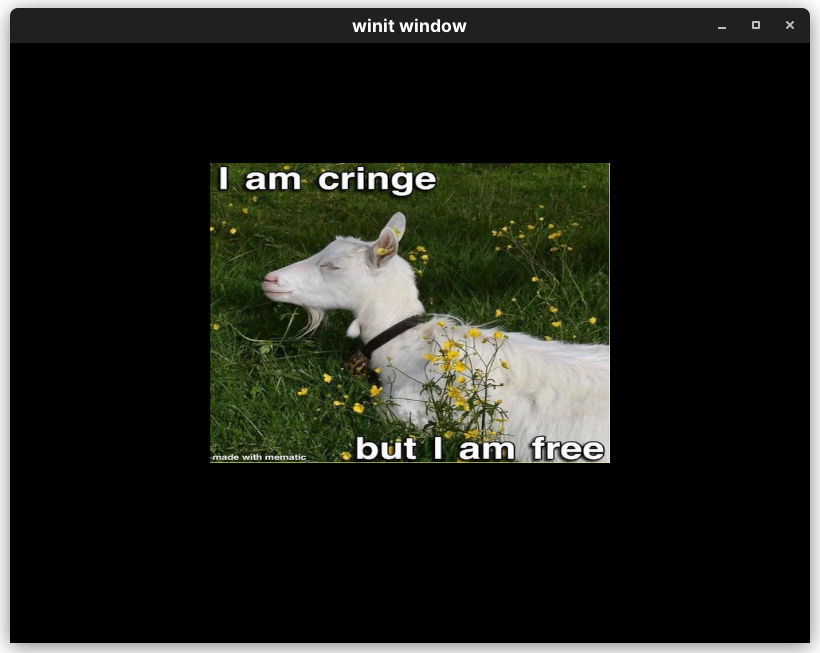
macOS:
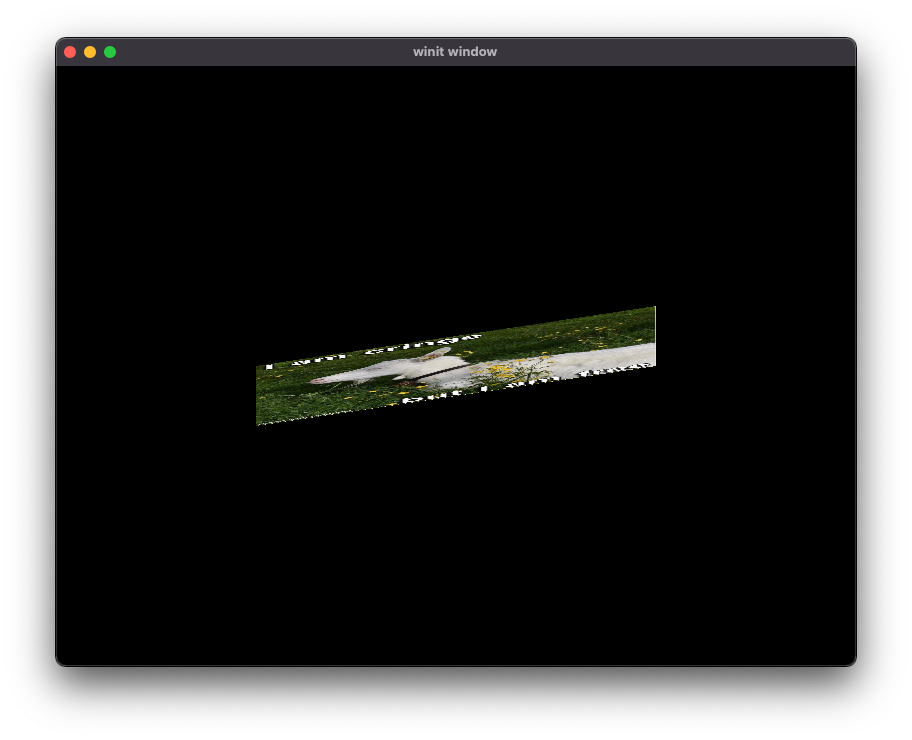
The transform matrix that I wrote:
How I'm converting this into bytes:
My WGSL shader:
The (vertex) shader that Vulkan sees, according to Nvidia Nsight (automatically decompiled to GLSL):
The matrix that Vulkan sees, according to Nvidia Nsight:
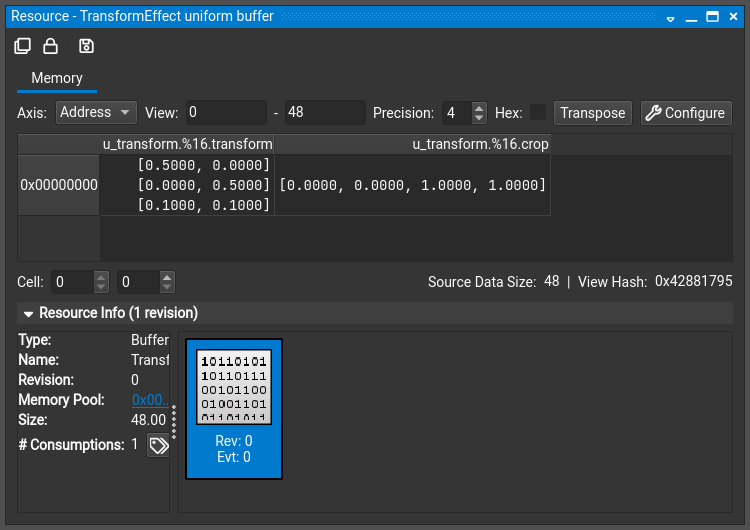
The shader that Metal sees, according to Xcode:
The text was updated successfully, but these errors were encountered: