-
-
Notifications
You must be signed in to change notification settings - Fork 85
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Bug: Scrolling breaks on Compose 1.3.0 in non-first child only #326
Comments
Hey! Thanks for such a detail explanation and the reproducer! This appears to be a bug in Compose. Specifically, the issue happens when Here is a simple reproducer. @Composable
fun Foo() {
val child1: @Composable (Modifier) -> Unit = remember { movableContentOf { modifier -> FixedScrollable(modifier) } }
val child2: @Composable (Modifier) -> Unit = remember { movableContentOf { modifier -> FixedScrollable(modifier) } }
var isHorizontal by remember { mutableStateOf(true) }
Column(modifier = Modifier.fillMaxSize()) {
val modifier = Modifier.fillMaxWidth().weight(1F)
if (isHorizontal) {
Row(modifier = modifier) {
child1(Modifier.weight(1F))
child2(Modifier.weight(1F))
}
} else {
Column(modifier = modifier) {
child1(Modifier.weight(1F))
child2(Modifier.weight(1F))
}
}
Button(onClick = { isHorizontal = !isHorizontal }) {
Text("Switch")
}
}
} I have filed JetBrains/compose-multiplatform#2696. I will also try to find a workaround. |
It doesn't look possible to fix it on Decompose side, as there seems no way to avoid |
Thank you @arkivanov for your swift reaction! I'll stay on |
Another possible workaround is to not use stack animations. |
I believe there is even better workaround:
|
Looks like the issue is fixed in JB Compose version |
Hey 👋
Intro & Affected Versions
I just realized that my bug that appeared when moving from Compose (JetBrains) version
1.2.2
to1.3.0
might be due to Decompose. It existed for quite a few1.3.0-rc*
versions. I am on Decompose1.0.0
(it already was a problem in1.0.0-beta-04
, possibly earlier). I have always setorg.jetbrains.kotlin:kotlin-gradle-plugin:1.7.20
for consistency.(A visual example and reproducer code is given at the end.)
Description
The scrollbar stops moving on the second child only (!) when on Compose
1.3.0
. In the case of non-lazy scrolling, even the content stops moving. This does not happen on the first child, which is why I think this is related to Decompose more than to JB Compose.Visuals
Click me
Expected Behavior (Compose
1.2.2
)Scrolling with the mouse wheel works too.
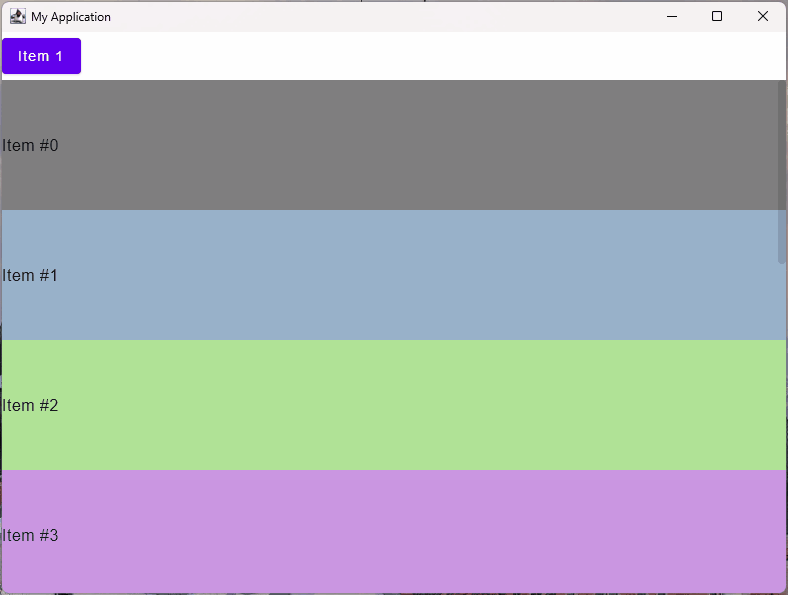
Wrong Behavior (Compose
1.3.0
) variantFixedScrollable
I also tried scrolling with the mouse wheel.
Wrong Behavior (Compose
1.3.0
) variantLazyScrollable
I also tried scrolling with the mouse wheel.
Reproducer
Click me
You can exchange occurrences of
FixedScrollable
andLazyScrollable
, both produce similar problems.The text was updated successfully, but these errors were encountered: