-
-
Notifications
You must be signed in to change notification settings - Fork 910
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[SUGGESTION] Autopot #491
Comments
An old ignored commit maybe you could use to help package minegame159.meteorclient.modules.player; import baritone.api.BaritoneAPI; import java.util.ArrayList; public class AutoPot extends Module {
private void startDrinking() {
private void drink() {
private void changeSlot(int slot) {
private boolean isNotPotion(ItemStack stack) { |
src/main/java/minegame159/meteorclient/modules/Modules.java private void initPlayer() {
|
All in #274 AUTOPOT v3 |
thanks for pasting in an entire java file rather than just linking it kek |
|
Same but worse than #600 |
Describe the feature

Automatically throws positive or damaging potions at yourself or other players.
It will have a delay option in settings as well as an option to choose what exact potion you want to throw.
Screenshots
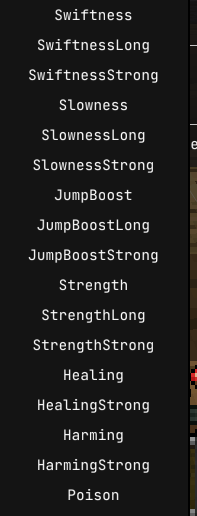
The text was updated successfully, but these errors were encountered: