diff --git a/README.md b/README.md index d4a54fc..4c9db8a 100644 --- a/README.md +++ b/README.md @@ -1,18 +1,19 @@ - # React Paginating [](https://travis-ci.org/ChoTotOSS/react-paginating) [](https://circleci.com/gh/davidnguyen179/react-paginating) [](https://dashboard.cypress.io/#/projects/qncx9e/runs) - [](https://img.shields.io/npm/dm/react-paginating.svg) [](https://codecov.io/gh/ChoTotOSS/react-paginating) [](https://badge.fury.io/js/react-paginating) [](https://github.com/ChoTotOSS/react-paginating/blob/master/LICENSE) [](http://makeapullrequest.com) [](https://unpkg.com/react-paginating@1.2.1/dist/) [](https://unpkg.com/react-paginating@1.2.1/dist/) [](https://greenkeeper.io/) [](https://packagequality.com/#?package=react-paginating) [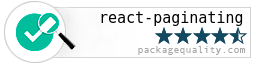](http://packagequality.com/#?package=react-paginating) -